Adăugarea numărului de postări personalizate în panoul de control WordPress
Panoul de control WordPress afișează în mod convenabil numărul de articole, pagini și comentarii. Aș dori să afișeze și numărul de articole, video-uri și desene animate (trei tipuri de postări personalizate înregistrate de tema mea). Cum pot include aceste informații în panoul "Right Now" de pe dashboard?
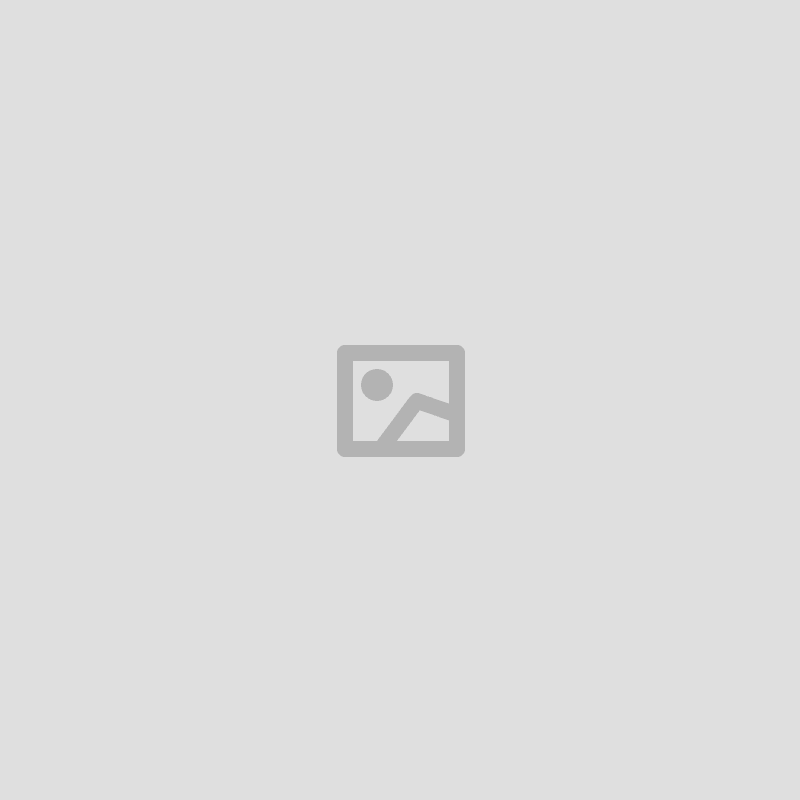
Da, există mai multe acțiuni în cadrul acelui widget, inclusiv right_now_content_table_end
. Exemplu:
function my_right_now() {
$num_widgets = wp_count_posts( 'widget' );
$num = number_format_i18n( $num_widgets->publish );
$text = _n( 'Widget', 'Widgets', $num_widgets->publish ); // _n() pentru singular/plural
if ( current_user_can( 'edit_pages' ) ) {
$num = "<a href='edit.php?post_type=widget'>$num</a>"; // Link pentru număr
$text = "<a href='edit.php?post_type=widget'>$text</a>"; // Link pentru text
}
echo '<tr>';
echo '<td class="first b b_pages">' . $num . '</td>'; // Celula cu număr
echo '<td class="t pages">' . $text . '</td>'; // Celula cu text
echo '</tr>';
}
add_action( 'right_now_content_table_end', 'my_right_now' ); // Adăugare acțiune
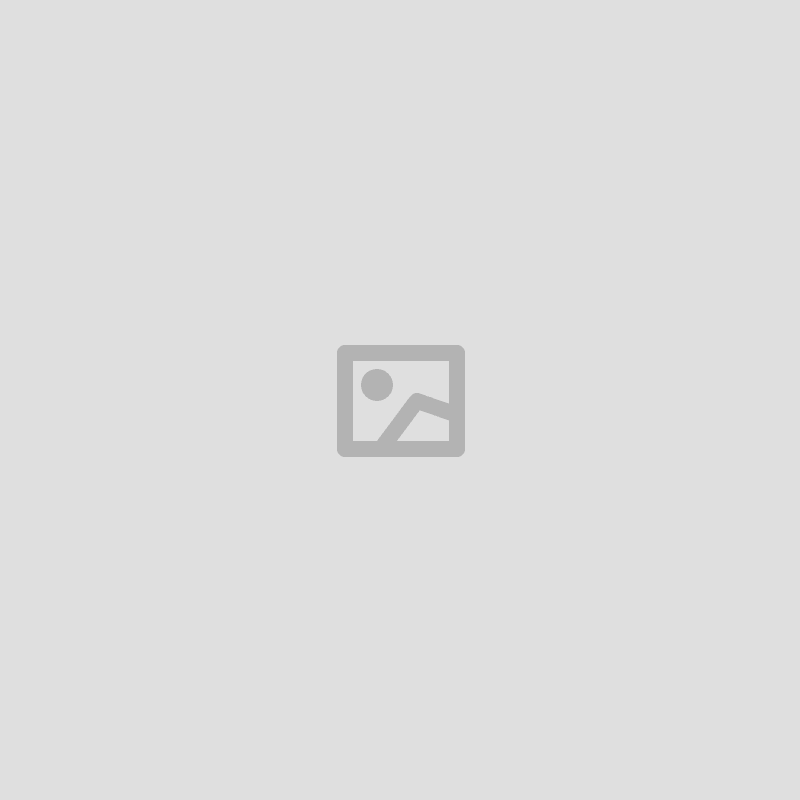
De asemenea, acoperit în cea mai bună colecție Wiki: http://wordpress.stackexchange.com/questions/1567/best-collection-of-code-for-your-functions-php-file
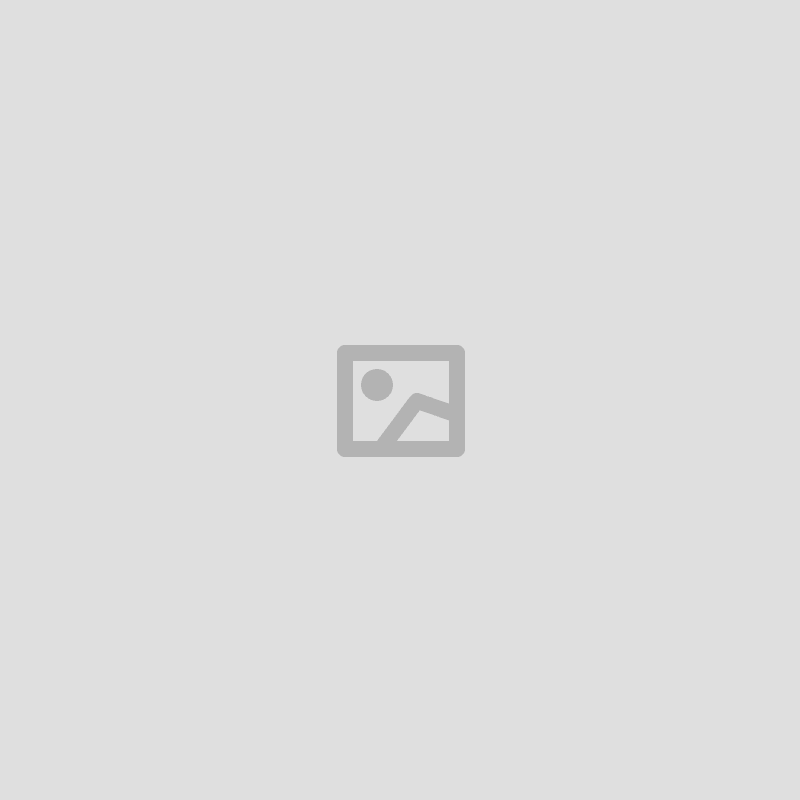
Bazându-ne pe același cod, puteți utiliza următoarea soluție pentru a afișa numărul de custom post types și taxonomii personalizate:
// Adaugă numărul de taxonomii și posturi personalizate în panoul de control
add_action( 'right_now_content_table_end', 'my_add_counts_to_dashboard' );
function my_add_counts_to_dashboard() {
// Numără taxonomiile personalizate
$taxonomies = get_taxonomies( array( '_builtin' => false ), 'objects' );
foreach ( $taxonomies as $taxonomy ) {
$num_terms = wp_count_terms( $taxonomy->name );
$num = number_format_i18n( $num_terms );
$text = _n( $taxonomy->labels->singular_name, $taxonomy->labels->name, $num_terms );
$associated_post_type = $taxonomy->object_type;
if ( current_user_can( 'manage_categories' ) ) {
$num = '<a href="edit-tags.php?taxonomy=' . $taxonomy->name . '&post_type=' . $associated_post_type[0] . '">' . $num . '</a>';
$text = '<a href="edit-tags.php?taxonomy=' . $taxonomy->name . '&post_type=' . $associated_post_type[0] . '">' . $text . '</a>';
}
echo '<td class="first b b-' . $taxonomy->name . 's">' . $num . '</td>';
echo '<td class="t ' . $taxonomy->name . 's">' . $text . '</td>';
echo '</tr><tr>';
}
// Numără posturile personalizate
$post_types = get_post_types( array( '_builtin' => false ), 'objects' );
foreach ( $post_types as $post_type ) {
$num_posts = wp_count_posts( $post_type->name );
$num = number_format_i18n( $num_posts->publish );
$text = _n( $post_type->labels->singular_name, $post_type->labels->name, $num_posts->publish );
if ( current_user_can( 'edit_posts' ) ) {
$num = '<a href="edit.php?post_type=' . $post_type->name . '">' . $num . '</a>';
$text = '<a href="edit.php?post_type=' . $post_type->name . '">' . $text . '</a>';
}
echo '<td class="first b b-' . $post_type->name . 's">' . $num . '</td>';
echo '<td class="t ' . $post_type->name . 's">' . $text . '</td>';
echo '</tr>';
if ( $num_posts->pending > 0 ) {
$num = number_format_i18n( $num_posts->pending );
$text = _n( $post_type->labels->singular_name . ' în așteptare', $post_type->labels->name . ' în așteptare', $num_posts->pending );
if ( current_user_can( 'edit_posts' ) ) {
$num = '<a href="edit.php?post_status=pending&post_type=' . $post_type->name . '">' . $num . '</a>';
$text = '<a href="edit.php?post_status=pending&post_type=' . $post_type->name . '">' . $text . '</a>';
}
echo '<td class="first b b-' . $post_type->name . 's">' . $num . '</td>';
echo '<td class="t ' . $post_type->name . 's">' . $text . '</td>';
echo '</tr>';
}
}
}
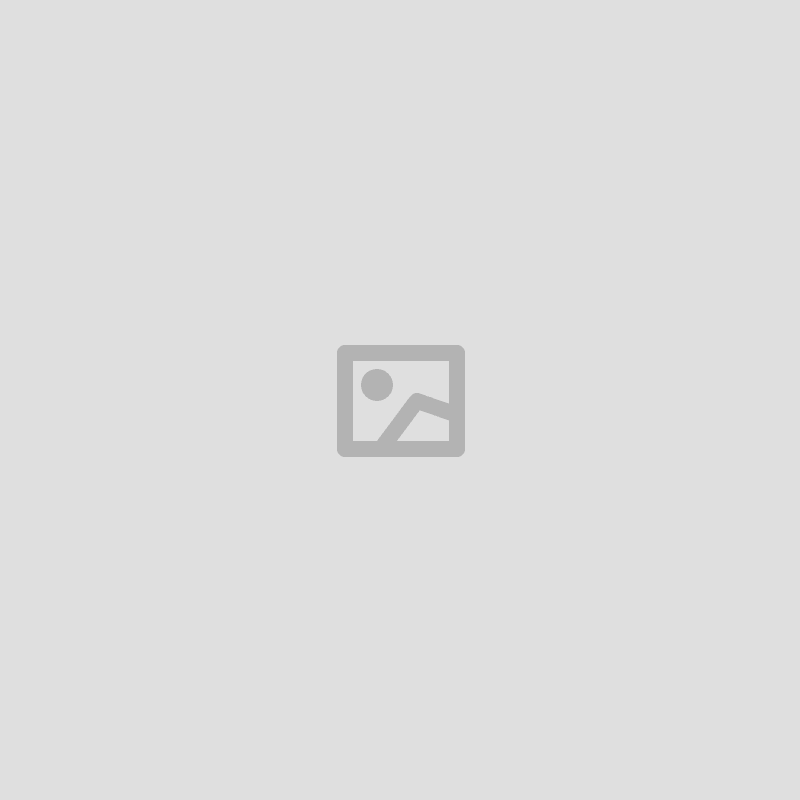
Pentru cei interesați să schimbe secțiunea "Discuție" pentru a afișa numărul de postări în așteptare în loc să le arate toate în "conținut", cârligul right_now_discussion_table_end poate fi folosit astfel:
add_action( 'right_now_discussion_table_end', 'my_add_counts_to_rightnow_discussion' );
function my_add_counts_to_rightnow_discussion() {
// Numără tipurile personalizate de postări
$post_types = get_post_types( array( '_builtin' => false, 'public' => true , 'show_ui' => true), 'objects' );
foreach ( $post_types as $post_type ) {
if ( current_user_can( 'edit_posts' ) ) {
$num_posts = wp_count_posts( $post_type->name );
$text = _n( $post_type->labels->singular_name, $post_type->labels->name, $num_posts->publish );
$num = number_format_i18n( $num_posts->pending );
$post_types = get_post_types( array( '_builtin' => false, 'public' => true , 'show_ui' => true), 'objects' );
$num = '<a href="edit.php?post_status=pending&post_type=' . $post_type->name . '">' . $num . '</a>';
$text = '<a class="waiting" href="edit.php?post_status=pending&post_type=' . $post_type->name . '">' . $text . ' În așteptare </a>';
echo '<td class="first b b-' . $post_type->name . 's">' . $num . '</td>';
echo '<td class="t ' . $post_type->name . 's">' . $text . '</td>';
echo '</tr>';
}
}
}
De asemenea, am eliminat condiția if(pending >0)
astfel încât tipurile de postări să se alinieze cu numărul din stânga. Dacă folosești acest cod, folosește codul lui Sébastien sau Adam pentru numărări și elimină secțiunea pentru postări în așteptare.
De notat, am adăugat o verificare pentru a vedea dacă tipurile de postări sunt publice și setate să fie afișate în interfața de utilizator. Aceasta poate fi adăugată la oricare dintre celelalte coduri.
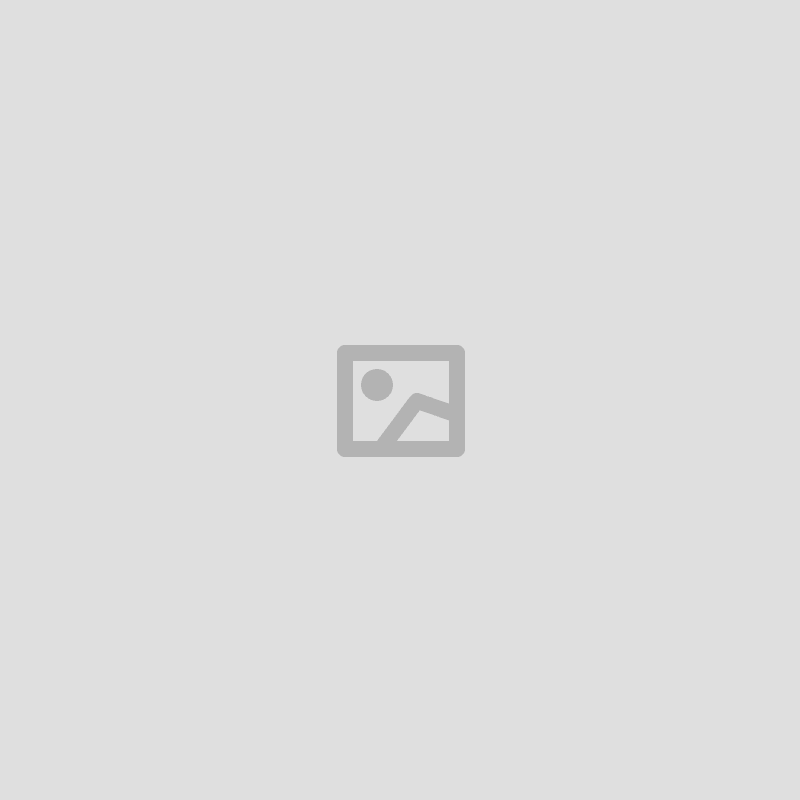
add_action( 'dashboard_glance_items', 'cor_right_now_content_table_end' );
function cor_right_now_content_table_end() {
$args = array(
'public' => true,
'_builtin' => false
);
$output = 'object';
$operator = 'and';
// Obține tipurile de postări personalizate
$post_types = get_post_types( $args, $output, $operator );
foreach ( $post_types as $post_type ) {
$num_posts = wp_count_posts( $post_type->name );
$num = number_format_i18n( $num_posts->publish );
$text = _n( $post_type->labels->singular_name, $post_type->labels->name, intval( $num_posts->publish ) );
if ( current_user_can( 'edit_posts' ) ) {
$output = '<a href="edit.php?post_type=' . $post_type->name . '">' . $num . ' ' . $text . '</a>';
}
echo '<li class="post-count ' . $post_type->name . '-count">' . $output . '</li>';
}
// Obține taxonomiile personalizate
$taxonomies = get_taxonomies( $args, $output, $operator );
foreach ( $taxonomies as $taxonomy ) {
$num_terms = wp_count_terms( $taxonomy->name );
$num = number_format_i18n( $num_terms );
$text = _n( $taxonomy->labels->singular_name, $taxonomy->labels->name, intval( $num_terms ) );
if ( current_user_can( 'manage_categories' ) ) {
$output = '<a href="edit-tags.php?taxonomy=' . $taxonomy->name . '">' . $num . ' ' . $text . '</a>';
}
echo '<li class="taxonomy-count ' . $taxonomy->name . '-count">' . $output . '</li>';
}
}
// Adaugă CSS personalizat pentru widget-ul "Într-o privire"
function custom_colors() {
echo '<style type="text/css">
.slides-count a:before {content:"\f233"!important}
.gallery-count a:before {content:"\f163"!important}
</style>';
}
add_action('admin_head', 'custom_colors');
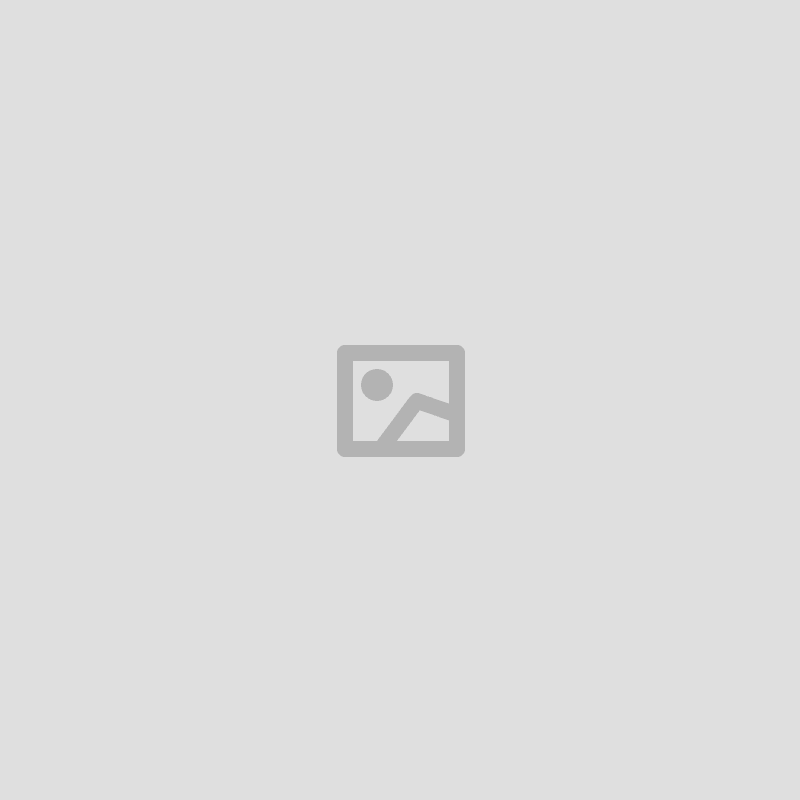