Obține postări cu ajax
Vreau să obțin postări cu ajax folosind funcția click.
Jquery
$(".get-posts").click(function(){
$.ajax({
type: 'POST',
url: '<?php echo admin_url('admin-ajax.php');?>',
data: { action : 'get_ajax_posts' },
success: function(response){
// Aici vom procesa răspunsul
}
});
});
Php
function get_ajax_posts() {
// Argumentele pentru interogare
$args = array(
'post_type' => array('products'),
'post_status' => array('publish'),
'posts_per_page' => 40,
'nopaging' => true,
'order' => 'DESC',
'orderby' => 'date',
'cat' => 1,
);
// Interogarea
$ajaxposts = new WP_Query( $args );
// Bucla
if ( $ajaxposts->have_posts() ) {
while ( $ajaxposts->have_posts() ) {
$ajaxposts->the_post();
get_template_part('products');
}
} else {
get_template_part('none');
}
/* Restaurăm datele originale ale postării */
wp_reset_postdata();
}
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
Sunt blocat aici.
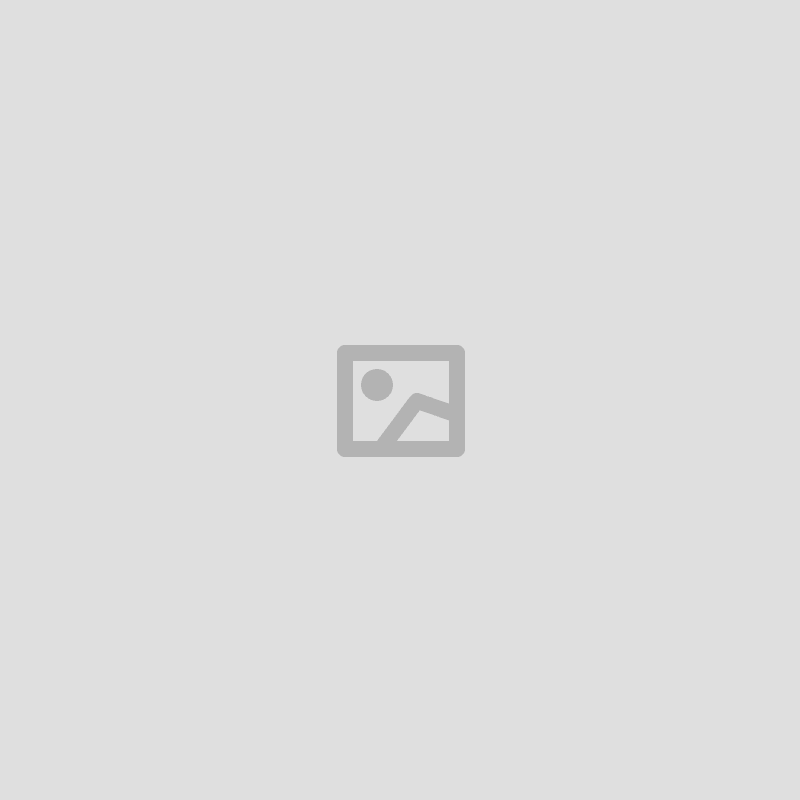
Opțiunea 1
Cea mai simplă metodă - returnează toate articolele în format JSON
și parcurge-le în JavaScript
PHP:
function get_ajax_posts() {
// Argumente pentru interogare
$args = array(
'post_type' => array('products'),
'post_status' => array('publish'),
'posts_per_page' => 40,
'nopaging' => true,
'order' => 'DESC',
'orderby' => 'date',
'cat' => 1,
);
// Interogarea
$ajaxposts = get_posts( $args ); // schimbat din wp_query în get_posts, deoarece `get_posts` returnează un array
echo json_encode( $ajaxposts );
exit; // încheie apelul AJAX (altfel va returna informații inutile în răspuns)
}
// Acțiune AJAX pentru utilizatorii autentificați și neautentificați
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
add_action('wp_ajax_nopriv_get_ajax_posts', 'get_ajax_posts');
JavaScript:
$.ajax({
type: 'POST',
url: '<?php echo admin_url('admin-ajax.php');?>',
dataType: "json", // adaugă tipul de date
data: { action : 'get_ajax_posts' },
success: function( response ) {
$.each( response, function( key, value ) {
console.log( key, value ); // acestea sunt datele articolelor.
} );
}
});
Opțiunea 2
Obține conținutul HTML și afișează-l pe ecran.
PHP:
function get_ajax_posts() {
// Argumente pentru interogare
$args = array(
'post_type' => array('products'),
'post_status' => array('publish'),
'posts_per_page' => 40,
'nopaging' => true,
'order' => 'DESC',
'orderby' => 'date',
'cat' => 1,
);
// Interogarea
$ajaxposts = new WP_Query( $args );
$response = '';
// Interogarea
if ( $ajaxposts->have_posts() ) {
while ( $ajaxposts->have_posts() ) {
$ajaxposts->the_post();
$response .= get_template_part('products');
}
} else {
$response .= get_template_part('none');
}
echo $response;
exit; // încheie apelul AJAX
}
// Acțiune AJAX pentru utilizatorii autentificați și neautentificați
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
add_action('wp_ajax_nopriv_get_ajax_posts', 'get_ajax_posts');
JavaScript:
$.ajax({
type: 'POST',
url: '<?php echo admin_url('admin-ajax.php');?>',
dataType: "html", // adaugă tipul de date
data: { action : 'get_ajax_posts' },
success: function( response ) {
console.log( response );
$( '.posts-area' ).html( response );
}
});
În al doilea exemplu, schimbă selectorul .posts-area
cu cel în care dorești să afișezi conținutul.
console.log
este folosit doar pentru a vedea informațiile returnate de apelul AJAX în consolă. Ar trebui să-l elimini după ce ai terminat.
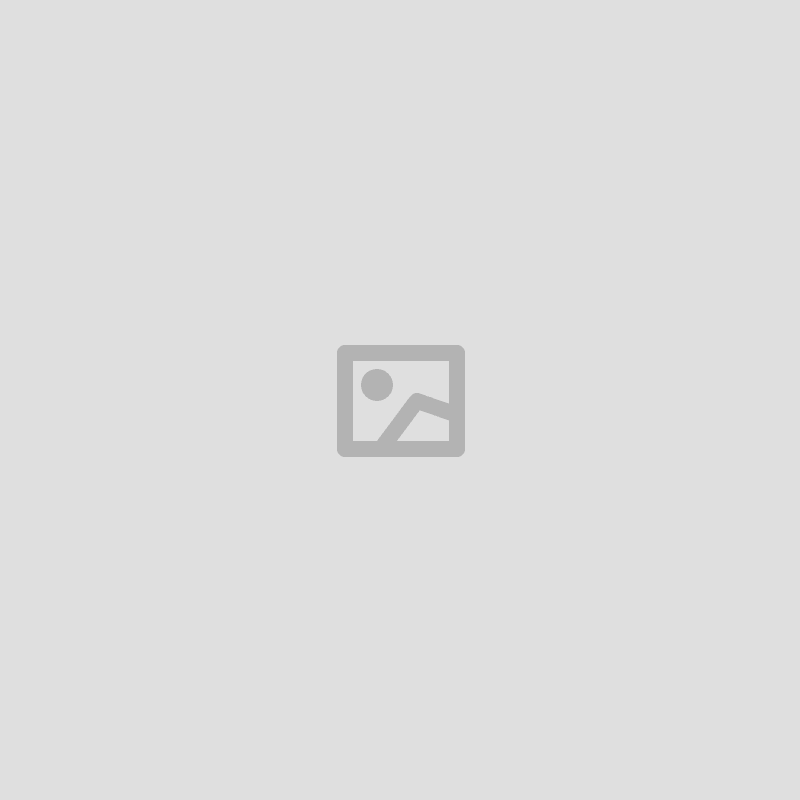
Mai mult, în cazul în care nu doriți să amestecați limbajele PHP și Javascript în aceleași fișiere (ceea ce nu ar trebui să faceți), puteți transmite URL-uri pentru cererile AJAX prin wp_localize_script (https://codex.wordpress.org/Function_Reference/wp_localize_script)
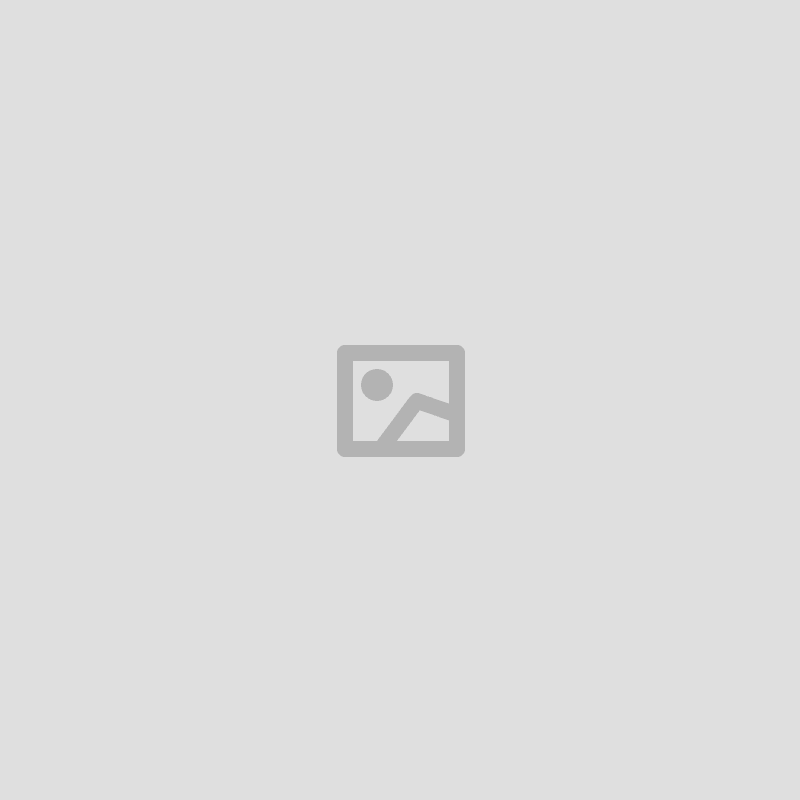
în opțiunea 1 există un new
eronat în linia $ajaxposts = new get_posts( $args );
ar trebui să scrie $ajaxposts = get_posts( $args );
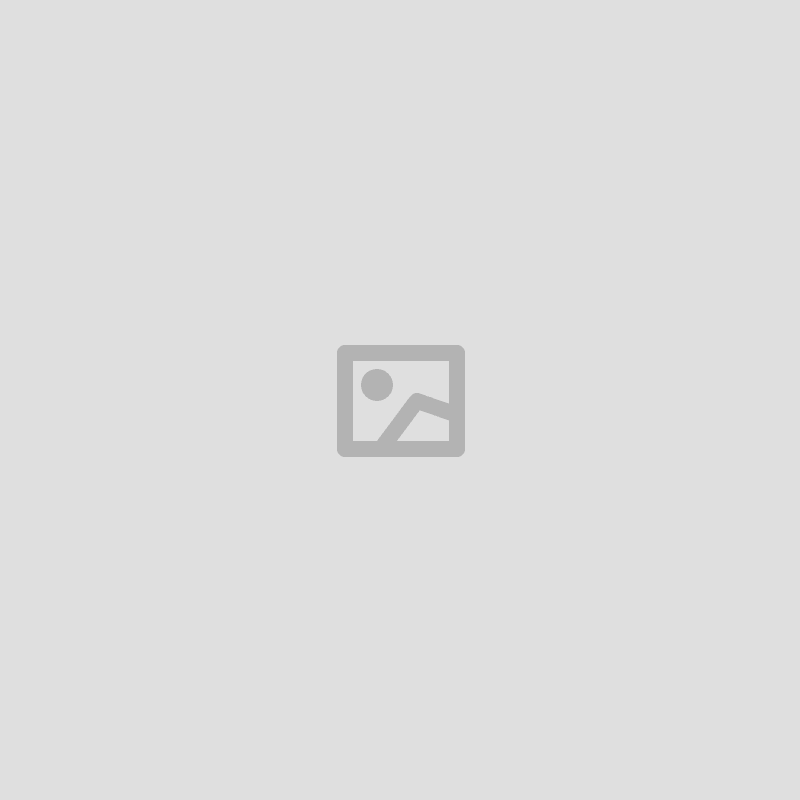