Ottenere i post con Ajax
Voglio ottenere i post con ajax tramite una funzione click.
jQuery
$(".get-posts").click(function(){
$.ajax({
type: 'POST',
url: '<?php echo admin_url('admin-ajax.php');?>',
data: { action : 'get_ajax_posts' },
success: function(response){
// Gestisci qui la risposta
}
});
});
PHP
function get_ajax_posts() {
// Argomenti della Query
$args = array(
'post_type' => array('products'),
'post_status' => array('publish'),
'posts_per_page' => 40,
'nopaging' => true,
'order' => 'DESC',
'orderby' => 'date',
'cat' => 1,
);
// La Query
$ajaxposts = new WP_Query( $args );
// Il Loop
if ( $ajaxposts->have_posts() ) {
while ( $ajaxposts->have_posts() ) {
$ajaxposts->the_post();
get_template_part('products');
}
} else {
get_template_part('none');
}
/* Ripristina i Dati Post Originali */
wp_reset_postdata();
}
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
Sono bloccato qui.
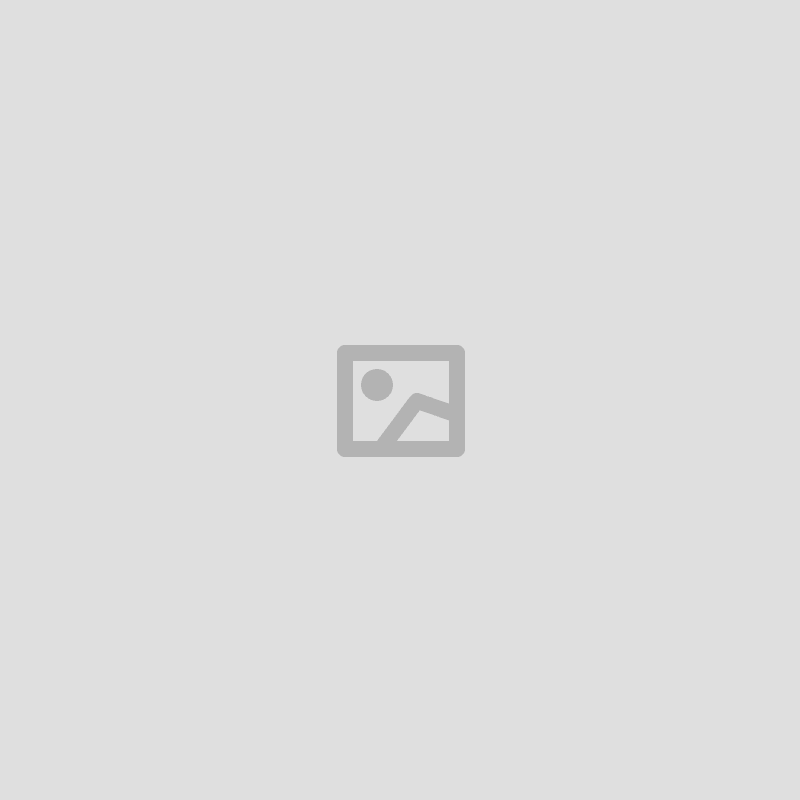
Opzione 1
Il modo semplice - restituire tutti i post in formato JSON
e ciclarli in JavaScript
PHP:
function get_ajax_posts() {
// Argomenti della Query
$args = array(
'post_type' => array('products'),
'post_status' => array('publish'),
'posts_per_page' => 40,
'nopaging' => true,
'order' => 'DESC',
'orderby' => 'date',
'cat' => 1,
);
// La Query
$ajaxposts = get_posts( $args ); // cambiato da wp_query a get_posts, perché `get_posts` restituisce un array
echo json_encode( $ajaxposts );
exit; // termina la chiamata AJAX (altrimenti restituirà informazioni inutili nella risposta)
}
// Azione AJAX per utenti loggati e non loggati
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
add_action('wp_ajax_nopriv_get_ajax_posts', 'get_ajax_posts');
JavaScript:
$.ajax({
type: 'POST',
url: '<?php echo admin_url('admin-ajax.php');?>',
dataType: "json", // aggiungi il tipo di dati
data: { action : 'get_ajax_posts' },
success: function( response ) {
$.each( response, function( key, value ) {
console.log( key, value ); // questi sono i dati dei post.
} );
}
});
Opzione 2
Ottieni il contenuto HTML e stampalo a schermo.
PHP:
function get_ajax_posts() {
// Argomenti della Query
$args = array(
'post_type' => array('products'),
'post_status' => array('publish'),
'posts_per_page' => 40,
'nopaging' => true,
'order' => 'DESC',
'orderby' => 'date',
'cat' => 1,
);
// La Query
$ajaxposts = new WP_Query( $args );
$response = '';
// La Query
if ( $ajaxposts->have_posts() ) {
while ( $ajaxposts->have_posts() ) {
$ajaxposts->the_post();
$response .= get_template_part('products');
}
} else {
$response .= get_template_part('none');
}
echo $response;
exit; // termina la chiamata AJAX
}
// Azione AJAX per utenti loggati e non loggati
add_action('wp_ajax_get_ajax_posts', 'get_ajax_posts');
add_action('wp_ajax_nopriv_get_ajax_posts', 'get_ajax_posts');
JavaScript:
$.ajax({
type: 'POST',
url: '<?php echo admin_url('admin-ajax.php');?>',
dataType: "html", // aggiungi il tipo di dati
data: { action : 'get_ajax_posts' },
success: function( response ) {
console.log( response );
$( '.posts-area' ).html( response );
}
});
Nel secondo esempio, cambia il selettore .posts-area
con quello in cui vuoi stampare il contenuto.
Il console.log
serve solo per vedere le informazioni restituite dalla chiamata AJAX nella console. Dovresti rimuoverlo una volta terminato.
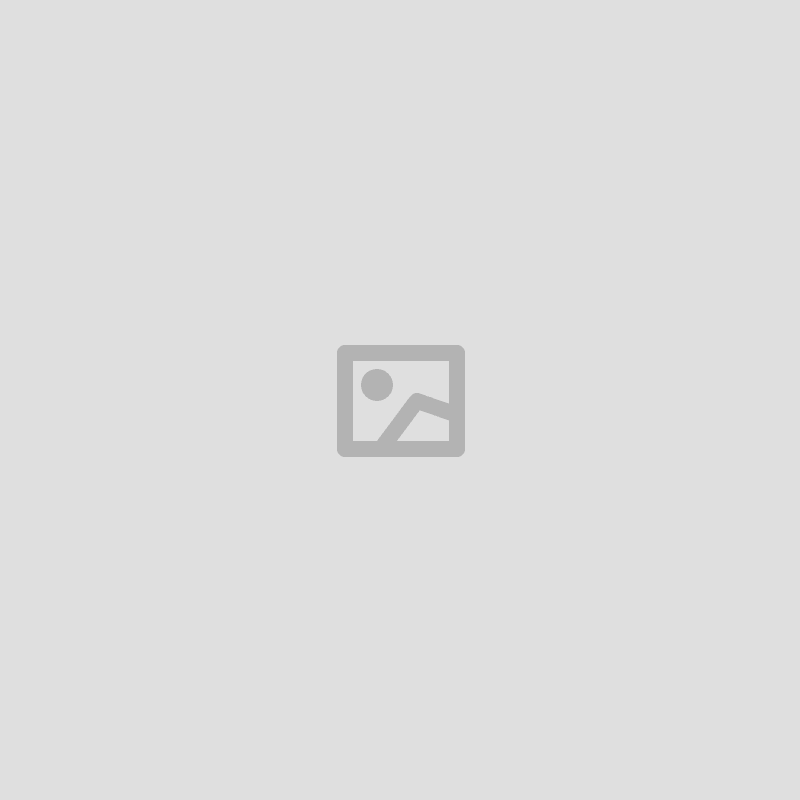
Inoltre, nel caso in cui non desideri mischiare linguaggi PHP e Javascript negli stessi file (cosa che dovresti evitare), puoi passare gli URL per le richieste AJAX utilizzando wp_localize_script (https://codex.wordpress.org/Function_Reference/wp_localize_script)
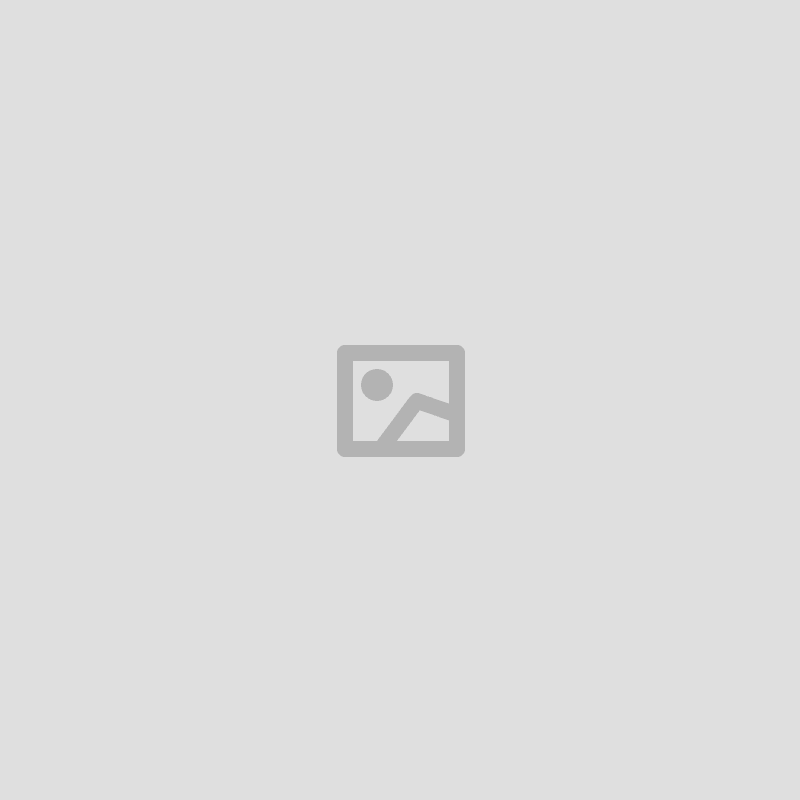
nell'opzione 1 c'è un errore con new
nella riga $ajaxposts = new get_posts( $args );
che dovrebbe invece essere $ajaxposts = get_posts( $args );
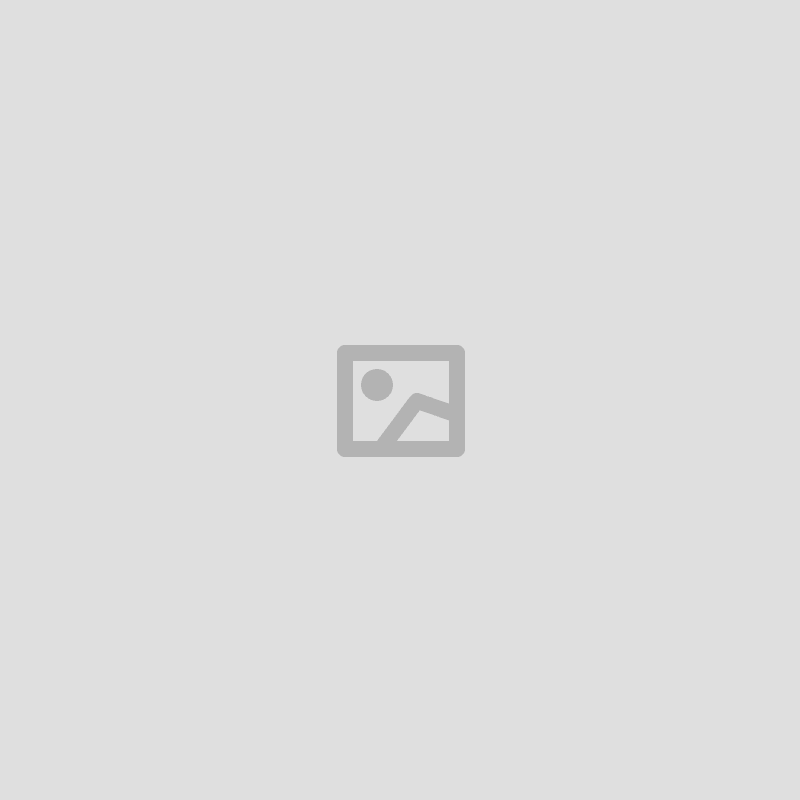