Come aggiungere una classe CSS a previous_post_link o ottenere l'URL del post precedente/successivo
Come posso aggiungere una classe CSS all'output di previous_post_link
o semplicemente ottenere l'URL e creare il markup HTML da solo
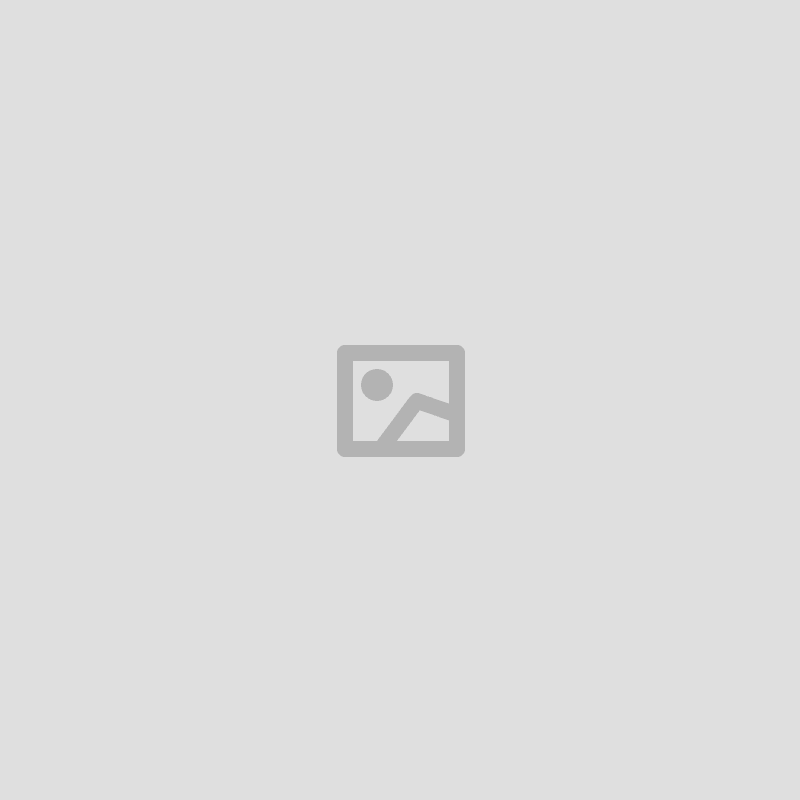
Esistono filtri per le funzioni previous_post_link e next_post_link che funzionano in modo diverso rispetto a previous_posts_link_attributes e next_posts_link_attributes, non sono sicuro del perché questo non sia documentato sul sito di WordPress.
function posts_link_next_class($format){
$format = str_replace('href=', 'class="next clean-gray" href=', $format);
return $format;
}
add_filter('next_post_link', 'posts_link_next_class');
function posts_link_prev_class($format) {
$format = str_replace('href=', 'class="prev clean-gray" href=', $format);
return $format;
}
add_filter('previous_post_link', 'posts_link_prev_class');
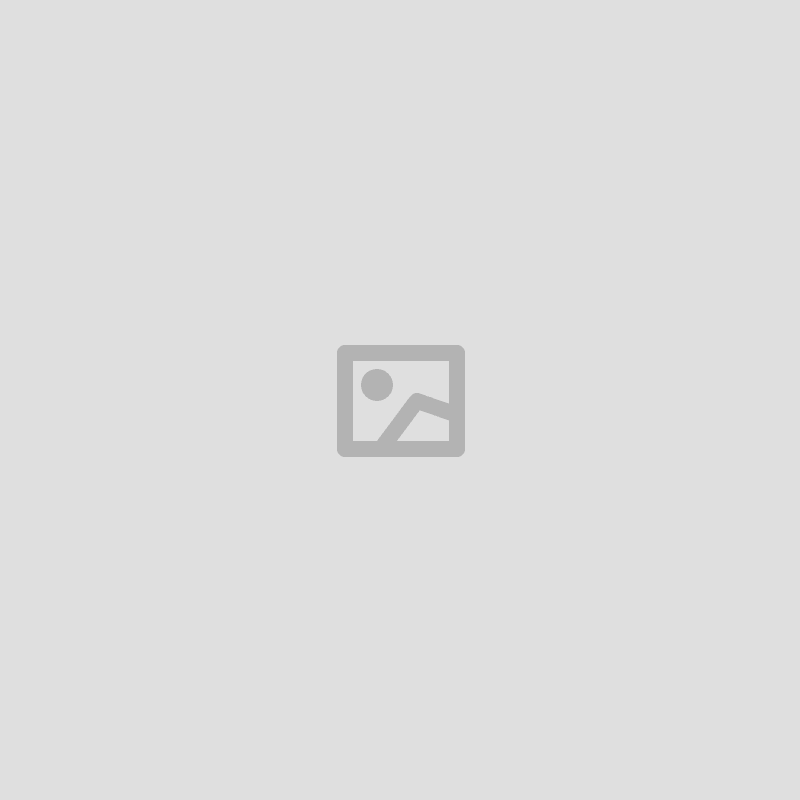
Puoi utilizzare la funzione più nativa che si trova "sotto" il previous_/next_post_link();
:
# get_adjacent_post( $in_same_cat = false, $excluded_categories = '', $previous = true )
$next_post_obj = get_adjacent_post( '', '', false );
$next_post_ID = isset( $next_post_obj->ID ) ? $next_post_obj->ID : '';
$next_post_link = get_permalink( $next_post_ID );
$next_post_title = '»'; // equivale a "»"
?>
<a href="<?php echo $next_post_link; ?>" rel="next" class="pagination pagination-link pagination-next">
<?php echo $next_post_title; ?>
</a>
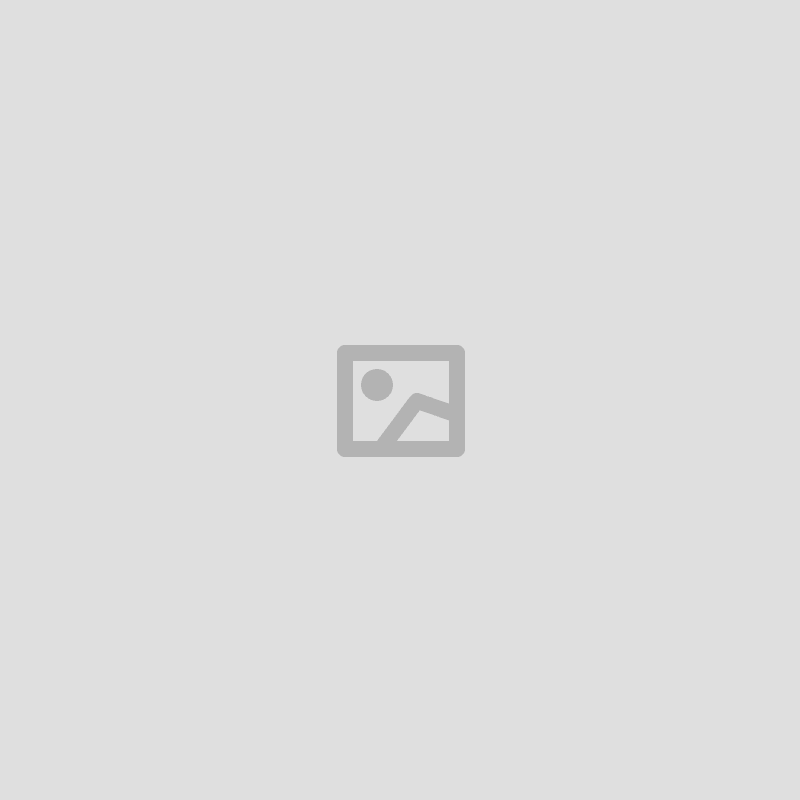
Il link alla tua sorgente GitHub è rotto. Potresti aggiornarlo per favore?
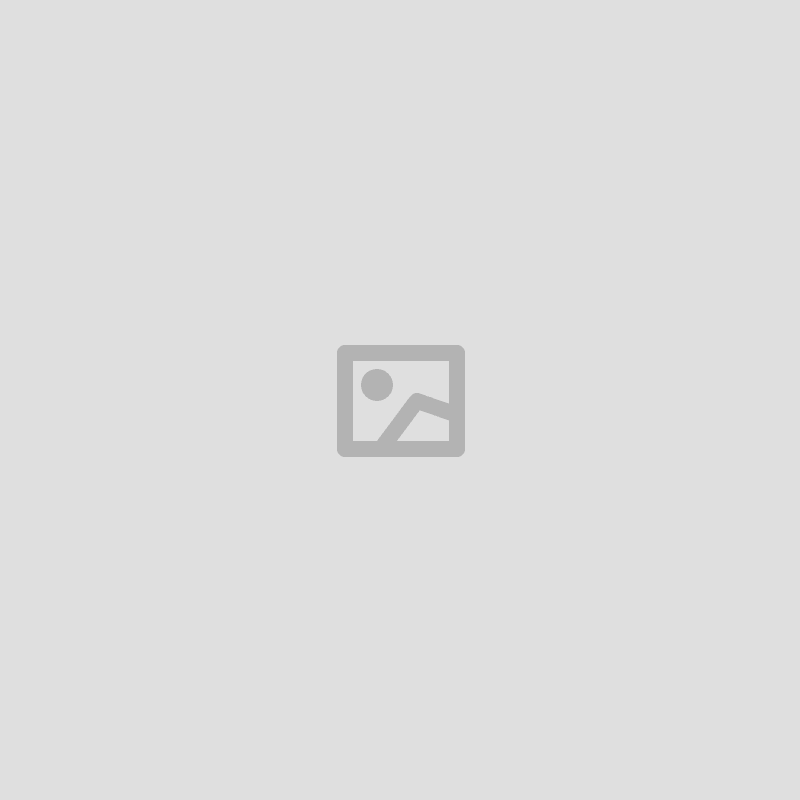
Un piccolo aggiunta: il problema con l'hook qui sotto è che sono nella forma plurale e la tua domanda implica che "vuoi stilizzarli nella forma singolare".
posts_link_attributes() {
return 'class="styled-button"';
}
add_filter('next_posts_link_attributes', 'posts_link_attributes');
add_filter('previous_posts_link_attributes', 'posts_link_attributes');
Sebbene funzionino perfettamente, questi sono i link che vengono visualizzati in fondo agli elenchi di post, non quelli in fondo ai singoli post. La logica direbbe che dovremmo poter duplicare questo codice con le forme singolari ("next_post_link" e "previous_post_link") e funzionerà. Sfortunatamente non è così.
Poiché i filtri per i link singoli next e previous vengono applicati in modo diverso vedi questo
Il codice qui sotto dovrebbe funzionare per i link singoli. Inseriscili nel tuo file function.php
function post_link_attributes($output) {
$code = 'class="styled-button"';
return str_replace('<a href=', '<a '.$code.' href=', $output);
}
add_filter('next_post_link', 'post_link_attributes');
add_filter('previous_post_link', 'post_link_attributes');
Il codice sopra è testato e funziona. Tuttavia puoi aggirare questo problema senza usare il function.php aggiungendo una classe LI intorno a ogni link:
<!--INIZIO: Navigazione Pagina-->
<?php if ( $wp_query->max_num_pages > 1 ) : // se c'è più di una pagina attiva la paginazione ?>
<nav id="page-nav">
<h1 class="hide">Navigazione Pagina</h1>
<ul class="clear-fix">
<li class="prev-link"><?php next_posts_link('« Pagina Precedente') ?></li>
<li class="next-link"><?php previous_posts_link('Pagina Successiva »') ?></li>
</ul>
</nav>
<?php endif; ?>
<!--FINE: Navigazione Pagina-->
Questo codice non è testato e nota la pluralità.
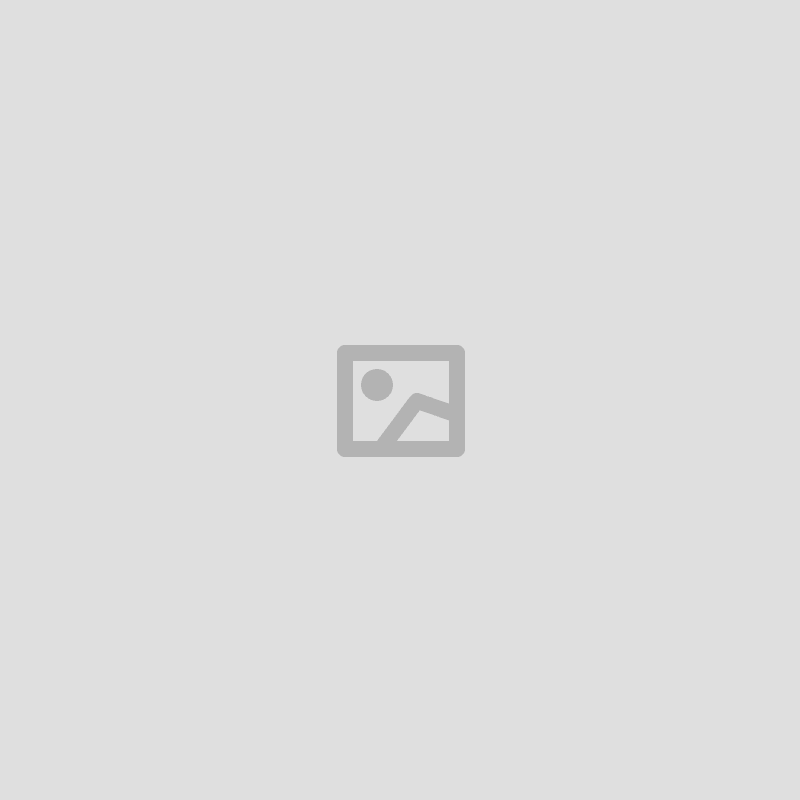
Prova nel seguente modo--
<?php $prv_post = get_previous_post();
$next_post = get_next_post();
?>
<?php if(!empty($prv_post)) { ?>
<a href="<?php echo get_permalink($prv_post->ID ); ?>" class="prev" rel="prev">
<span class="meta-nav"><?php _e('Post Precedente', 'awe') ?></span>
<span class="nav-icon"><i class="fa fa-angle-double-left"></i></span>
<?php echo get_the_title($prv_post->ID ); ?> ...
</a>
<?php } ?>
<?php if(!empty($next_post)) { ?>
<a href="<?php echo get_permalink($next_post->ID ); ?>" class="next" rel="next">
<span class="meta-nav"><?php _e('Post Successivo', 'awe') ?></span>
<span class="nav-icon"><i class="fa fa-angle-double-right"></i></span>
<?php echo get_the_title($next_post->ID ); ?> ...
</a>
<?php } ?>
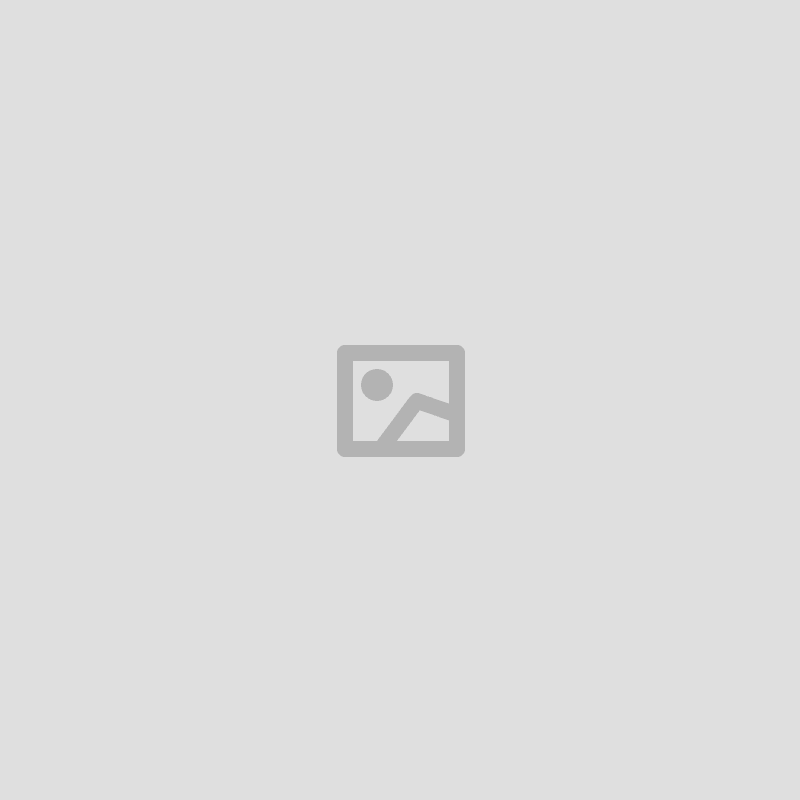
Perché stai usando echo get_*
, quando ci sono funzioni correlate che emettono l'output di default, come the_title()
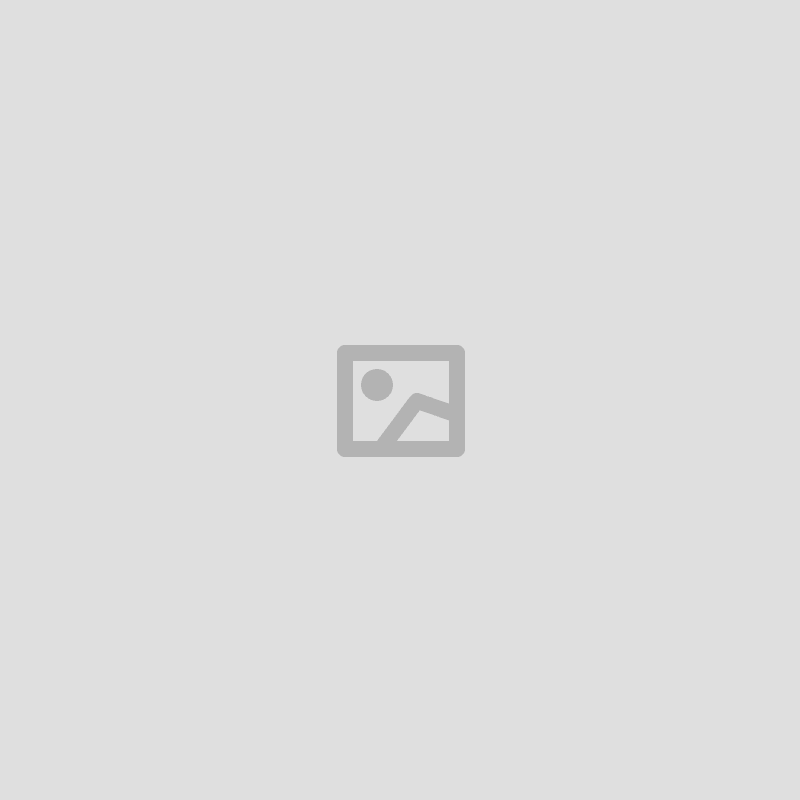
Tutto molto buono, anche se il modo più semplice che ho trovato è stato aggiungere un div nel file functions.php che avvolge i link. Ho chiamato il mio class="plinks"
if ( ! function_exists( 'themename_post_nav' ) ) :
function themename_post_nav() {
global $post;
$previous = ( is_attachment() ) ? get_post( $post->post_parent ) : get_adjacent_post( false, '', true );
$next = get_adjacent_post( false, '', false );
if ( ! $next && ! $previous )
return;
?>
<nav>
<div class="plinks">
<?php next_post_link( '%link', _x( 'Precedente', 'Link precedente', 'themename' ) ); ?>
<?php previous_post_link( '%link', _x( 'Successivo', 'Link successivo', 'themename' ) ); ?>
</div>
</nav>
<?php
}
endif;
poi nel tuo file css basta scrivere una nuova classe per
.plinks a{
display:inline-block;
margin:1em 4px;
font-size:1em;
font-weight:500;
border:0;
padding:8px 1em;
color:#fff;
background:#000;
}
.plinks a:hover{
opacity:0.8;
}
Chiamalo in qualsiasi pagina template del tuo tema usando
<?php themename_post_nav(); ?>
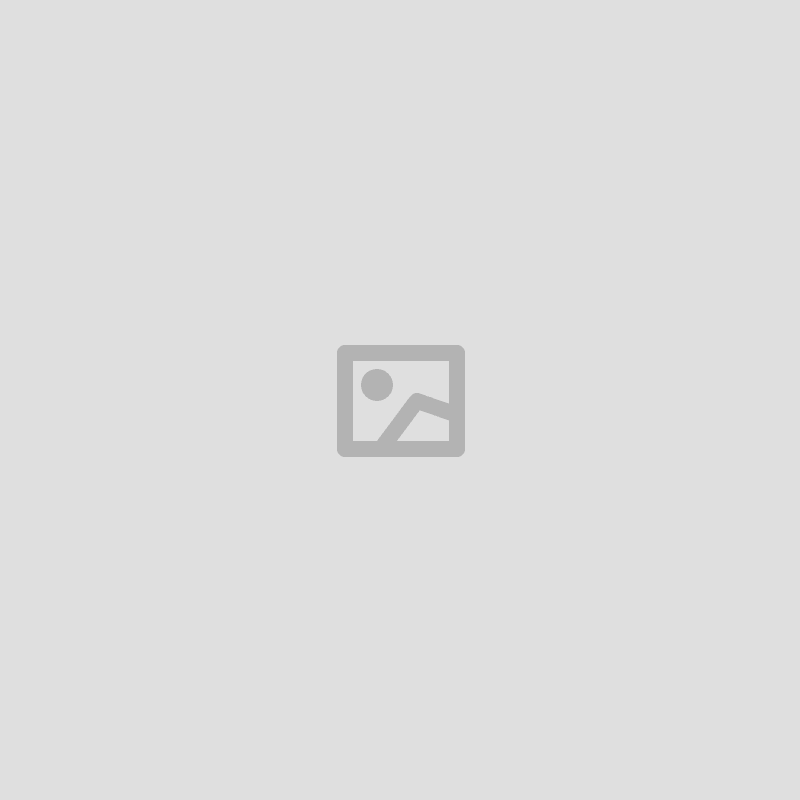
Questo codice verificherà se le variabili $previous_post_obj e $next_post_obj sono vuote prima di generare i pulsanti "precedente" e "successivo", rispettivamente. Se una delle variabili è vuota, il corrispondente pulsante non verrà visualizzato.
<div>
<?php $previous_post_obj = get_adjacent_post( '', '', true );
if ( ! empty( $previous_post_obj ) ) {
$previous_post_ID = isset( $previous_post_obj->ID ) ? $previous_post_obj->ID : '';
$previous_post_link = get_permalink( $previous_post_ID ); ?>
<a href="<?php echo $previous_post_link; ?>" rel="prev" class="prev">
<span>precedente</span>
</a>
<?php } ?>
<?php $next_post_obj = get_adjacent_post( '', '', false );
if ( ! empty( $next_post_obj ) ) {
$next_post_ID = isset( $next_post_obj->ID ) ? $next_post_obj->ID : '';
$next_post_link = get_permalink( $next_post_ID ); ?>
<a href="<?php echo $next_post_link; ?>" rel="next" class="next">
<span>successivo</span>
</a>
<?php } ?>
</div>
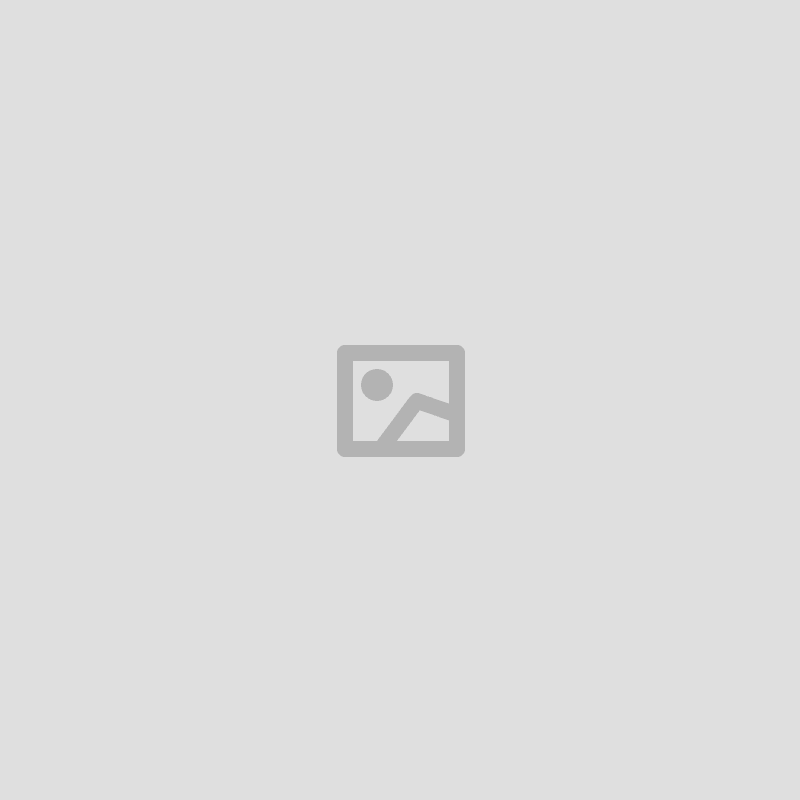