Escludere l'ID di un articolo da wp_query
Come posso escludere uno specifico articolo da una query WP_Query? (Per esempio, mostrare tutti gli articoli tranne quello con ID 278)
Ho provato l'argomento post__not_in ma rimuove tutti gli articoli..
Ogni aiuto sarebbe apprezzato.
Ecco la mia query attuale
<?php
$temp = $wp_query;
$wp_query= null;
$wp_query = new WP_Query(array(
'post_type' => 'case-study',
'paged' => $paged,
));
while ($wp_query->have_posts()) : $wp_query->the_post();
?>
Grazie
Il parametro che stai cercando è post__not_in
(kaiser ha un errore di battitura nella sua risposta). Quindi il codice potrebbe essere:
<?php
$my_query = new WP_Query(array(
'post__not_in' => array(278),
'post_type' => 'case-study',
'paged' => $paged,
));
while ($my_query->have_posts()) : $my_query->the_post(); endwhile;
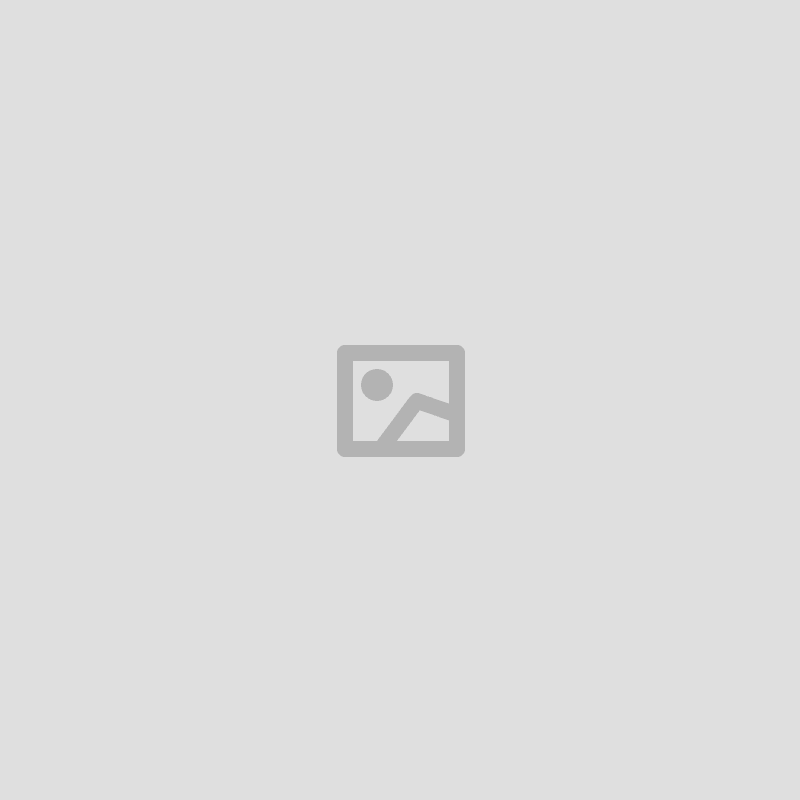
@Ziki la virgola nell'array non è un errore di battitura, è una sintassi PHP valida, se è questo che intendi.
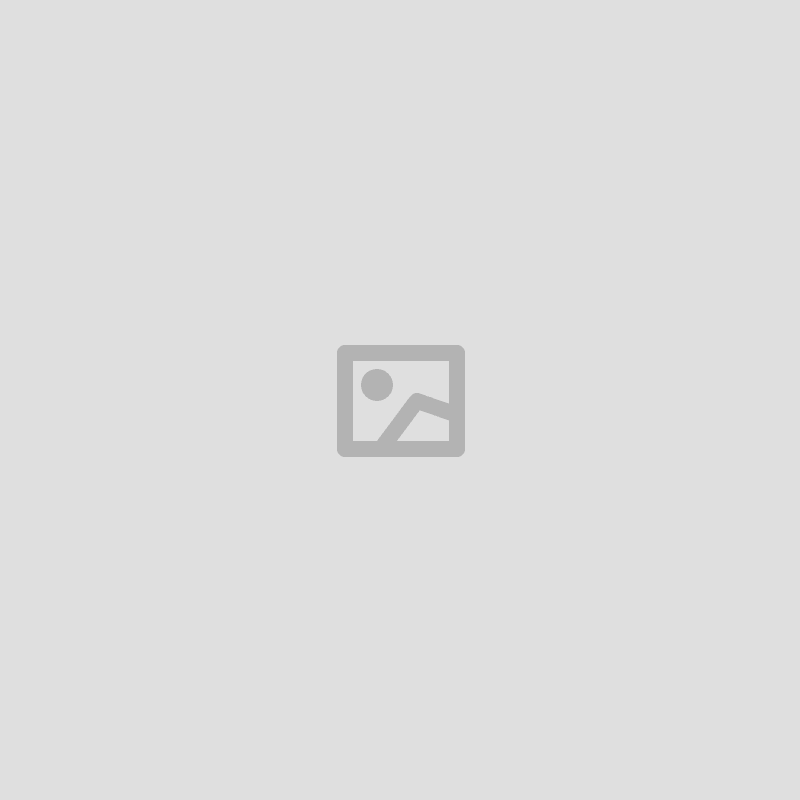
@leonziyo - no, originariamente aveva scritto "posts__not_in" invece di "post__not_in", vedi la cronologia della sua risposta. La virgola è corretta
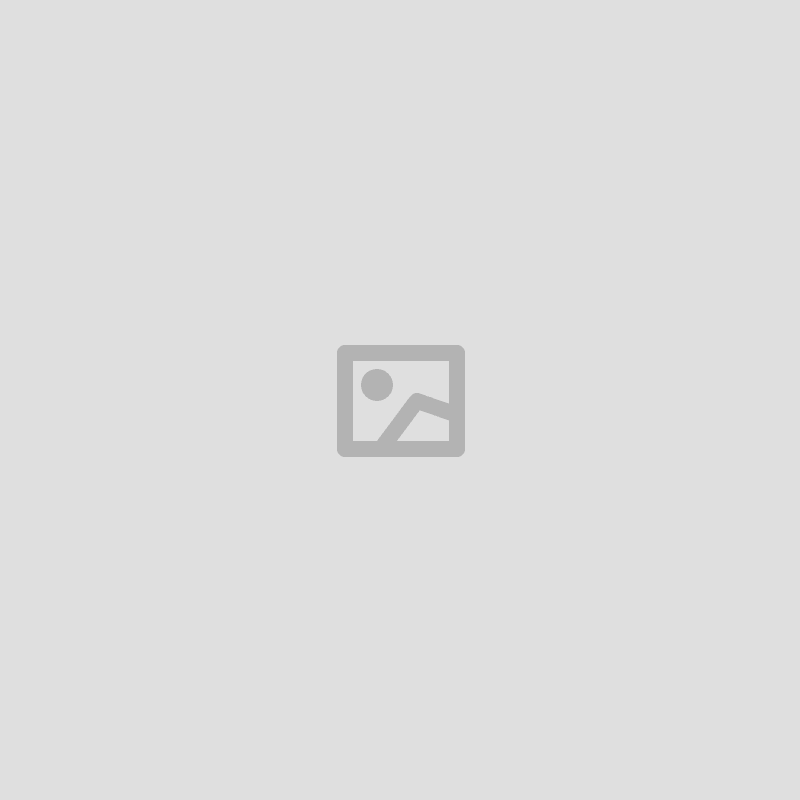
Devi definire l'argomento post__not_in
come array. Anche per un singolo valore. E per favore non sovrascrivere le variabili globali del core con elementi temporanei.
<?php
$query = new WP_Query( array(
'post_type' => 'case-study',
'paged' => $paged,
'post__not_in' => array( 1, ),
) );
if ( $query->have_posts() ) {
while ( $query->have_posts() ) {
$query->the_post();
// fai qualcosa
} // endwhile;
} // endif;
?>
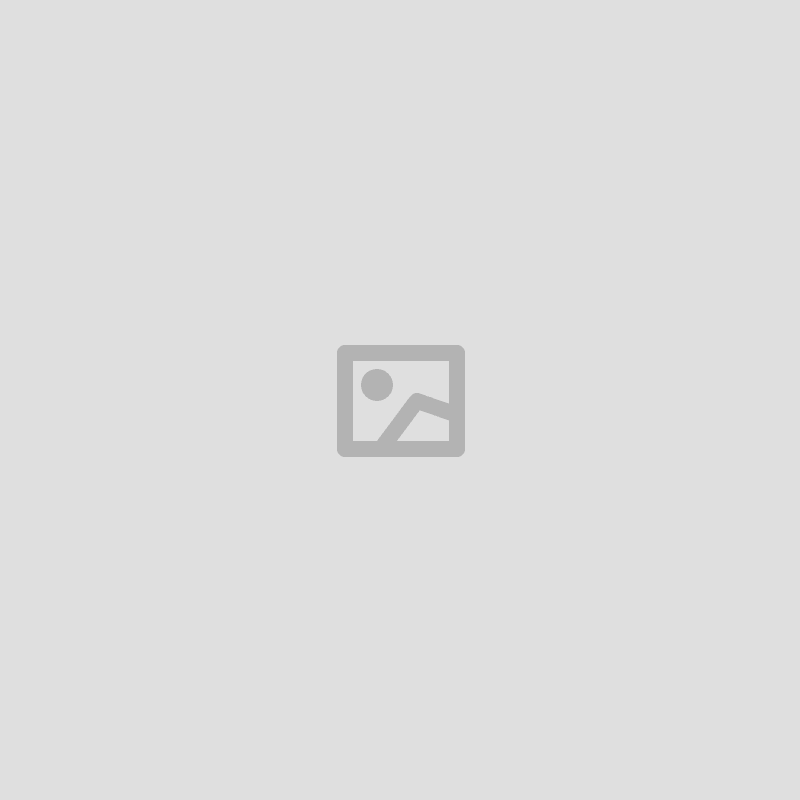
Suppongo che questo fosse complesso, ma per rispondere alla tua domanda originale, ho raccolto tutti gli ID dei post in un array nel primo ciclo, ed escluso quei post dal secondo ciclo utilizzando 'post__not_in' che si aspetta un array di ID dei post
<?php
$args1 = array('category_name' => 'test-cat-1', 'order' => 'ASC');
$q1 = new WP_query($args);
if($q1->have_posts()) :
$firstPosts = array();
while($q1->have_posts()) : $q1->the_post();
$firstPosts[] = $post->ID; // aggiunge l'ID del post all'array
echo '<div class="item">';
echo "<h2>" . get_the_title() . "</h2>";
echo "</div>";
endwhile;
endif;
/****************************************************************************/
// l'array di ID dei post raccolti nel primo ciclo può ora essere usato come valore per il parametro 'post__not_in' nel secondo ciclo
$args2 = array('post__not_in' => $firstPosts, 'order' => 'ASC' );
$q2 = new WP_query($args2);
if($q2->have_posts()) :
while($q2->have_posts()) : $q2->the_post();
echo '<div class="item">';
echo "<h2>" . get_the_title() . "</h2>";
echo "</div>";
endwhile;
endif;
?>
Il primo ciclo mostra tutti i post in una categoria e raccoglie gli ID dei post in un array.
Il secondo ciclo mostra tutti i post, escludendo quelli del primo ciclo.
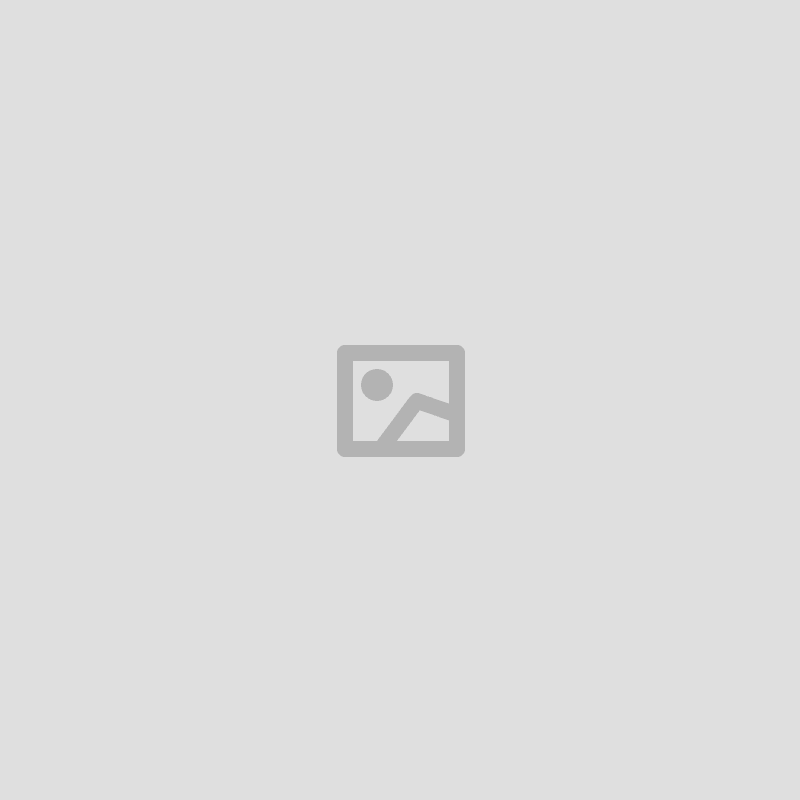
Codici alternativi;
Escludi post da categoria
<?php
add_action('pre_get_posts', 'exclude_category_posts');
function exclude_category_posts( $query ) {
// Esclude le categorie con ID 22 e 27 dalla homepage
if($query->is_main_query() && $query->is_home()) {
$query->set('cat', array( -22, -27 ));
}
}
?>
Rimuovi post dalla pagina homepage
<?php
add_action('pre_get_posts', 'wpsites_remove_posts_from_home_page');
function wpsites_remove_posts_from_home_page( $query ) {
// Rimuove i post dalle categorie con ID 1 e 11 dalla homepage
if($query->is_main_query() && $query->is_home()) {
$query->set('category__not_in', array(-1, -11));
}
}
?>
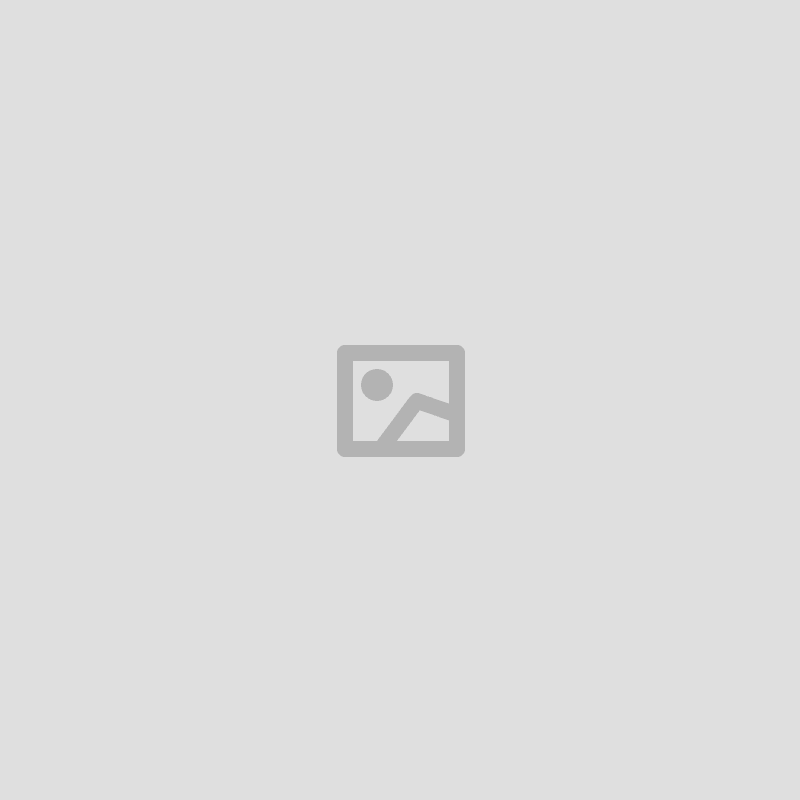