Ricerca live ajax per titoli degli articoli
Sto creando una ricerca live con AJAX per filtrare i titoli degli articoli nel mio tema WordPress. Quando scrivo qualcosa nell'input vengono mostrati tutti gli articoli nei risultati, senza filtrare per ottenere articoli personalizzati.
Come effettuare una ricerca live e trovare un articolo con AJAX?
Function.php
<?php
// Aggiungi azioni per gli utenti loggati e non loggati
add_action('wp_ajax_my_action' , 'data_fetch');
add_action('wp_ajax_nopriv_my_action','data_fetch');
function data_fetch(){
$the_query = new WP_Query(array('post_per_page'=>5));
if( $the_query->have_posts() ) :
while( $the_query->have_posts() ): $the_query->the_post(); ?>
<h2><a href="<?php echo esc_url( post_permalink() ); ?>"><?php the_title();?></a></h2>
<?php endwhile;
wp_reset_postdata();
endif;
die();
}?>
Script:
<script>
function fetch(){
// Invia richiesta AJAX all'endpoint di WordPress
$.post('<?php echo admin_url('admin-ajax.php'); ?>',{'action':'my_action'},
function(response){
$('#datafetch').append(response);
console.log(result);
});
}
</script>
HTML:
<input type="text" onkeyup="fetch()"></input>
<div id="datafetch">
</div>
Ecco una soluzione funzionante (testata così com'è)
L'HTML (potrebbe far parte del contenuto della pagina)
<input type="text" name="keyword" id="keyword" onkeyup="fetch()"></input>
<div id="datafetch">I risultati della ricerca appariranno qui</div>
Il JavaScript (va nel file functions.php del tuo tema)
// aggiungi il JavaScript per l'AJAX fetch
add_action( 'wp_footer', 'ajax_fetch' );
function ajax_fetch() {
?>
<script type="text/javascript">
function fetch(){
jQuery.ajax({
url: '<?php echo admin_url('admin-ajax.php'); ?>',
type: 'post',
data: { action: 'data_fetch', keyword: jQuery('#keyword').val() },
success: function(data) {
jQuery('#datafetch').html( data );
}
});
}
</script>
<?php
}
E infine la chiamata AJAX va nel file functions.php del tuo tema
// la funzione AJAX
add_action('wp_ajax_data_fetch' , 'data_fetch');
add_action('wp_ajax_nopriv_data_fetch','data_fetch');
function data_fetch(){
$the_query = new WP_Query( array( 'posts_per_page' => -1, 's' => esc_attr( $_POST['keyword'] ), 'post_type' => 'post' ) );
if( $the_query->have_posts() ) :
while( $the_query->have_posts() ): $the_query->the_post(); ?>
<h2><a href="<?php echo esc_url( get_permalink() ); ?>"><?php the_title();?></a></h2>
<?php endwhile;
wp_reset_postdata();
endif;
die();
}
Aggiunta: Ecco come ho reso più precisa la mia funzione AJAX di ricerca:
// la funzione AJAX
add_action('wp_ajax_data_fetch' , 'data_fetch');
add_action('wp_ajax_nopriv_data_fetch','data_fetch');
function data_fetch(){
$the_query = new WP_Query(
array(
'posts_per_page' => -1,
's' => esc_attr( $_POST['keyword'] ),
'post_type' => 'post'
)
);
if( $the_query->have_posts() ) :
while( $the_query->have_posts() ): $the_query->the_post();
$myquery = esc_attr( $_POST['keyword'] );
$a = $myquery;
$search = get_the_title();
if( stripos("/{$search}/", $a) !== false) {?>
<h4><a href="<?php echo esc_url( get_permalink() ); ?>"><?php the_title();?></a></h4>
<?php
}
endwhile;
wp_reset_postdata();
endif;
die();
}
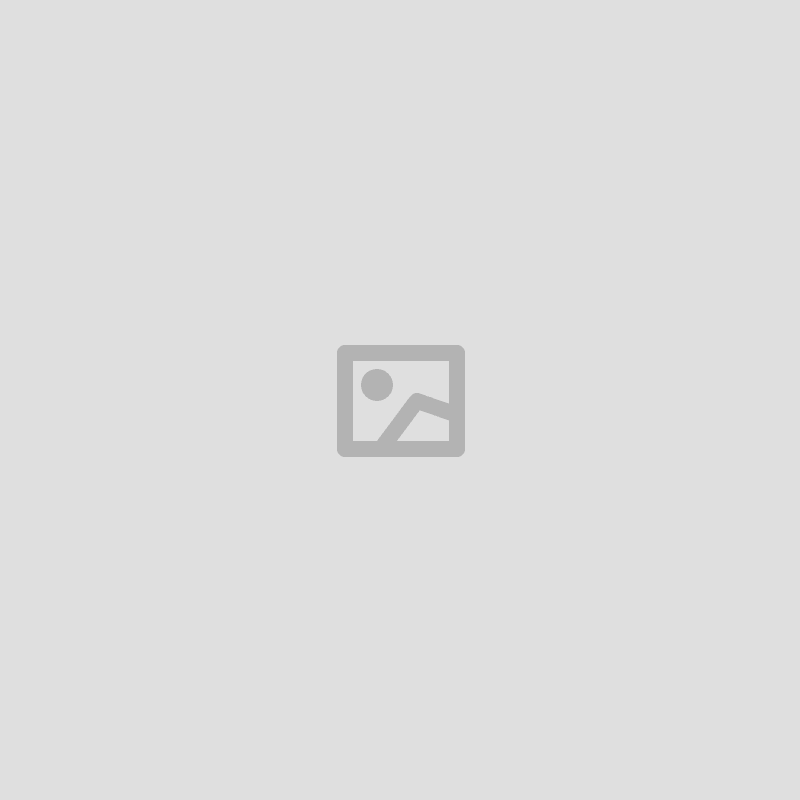
Conosci un modo per cercare la parola chiave solo nel titolo o solo nella descrizione?
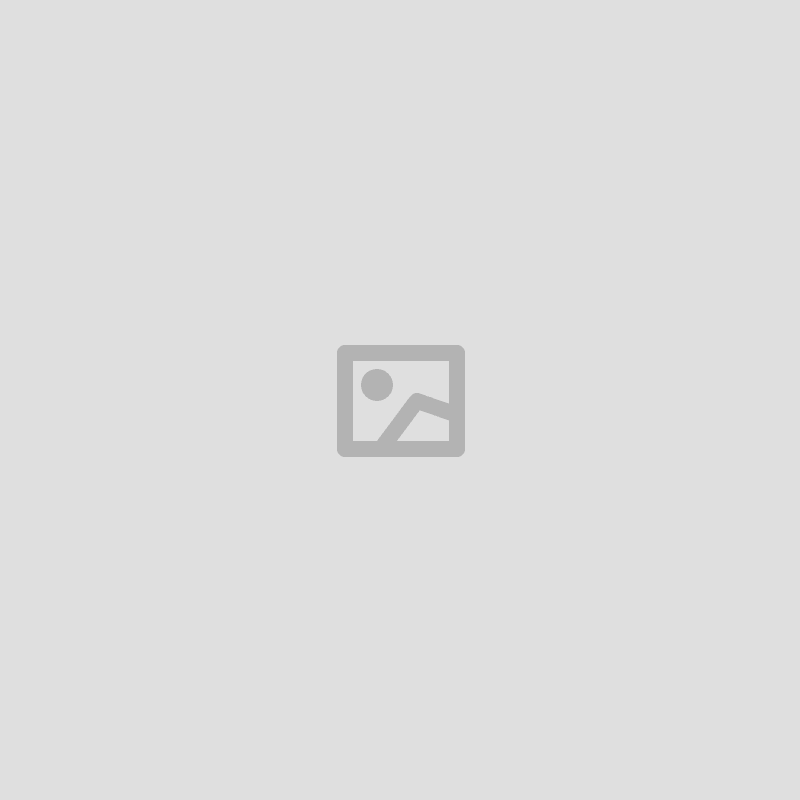
magari utilizzando solo il filtro posts_where
invece di 's'
come in https://wordpress.stackexchange.com/questions/298888/wp-query-where-title-begins-with-a-specific-letter)
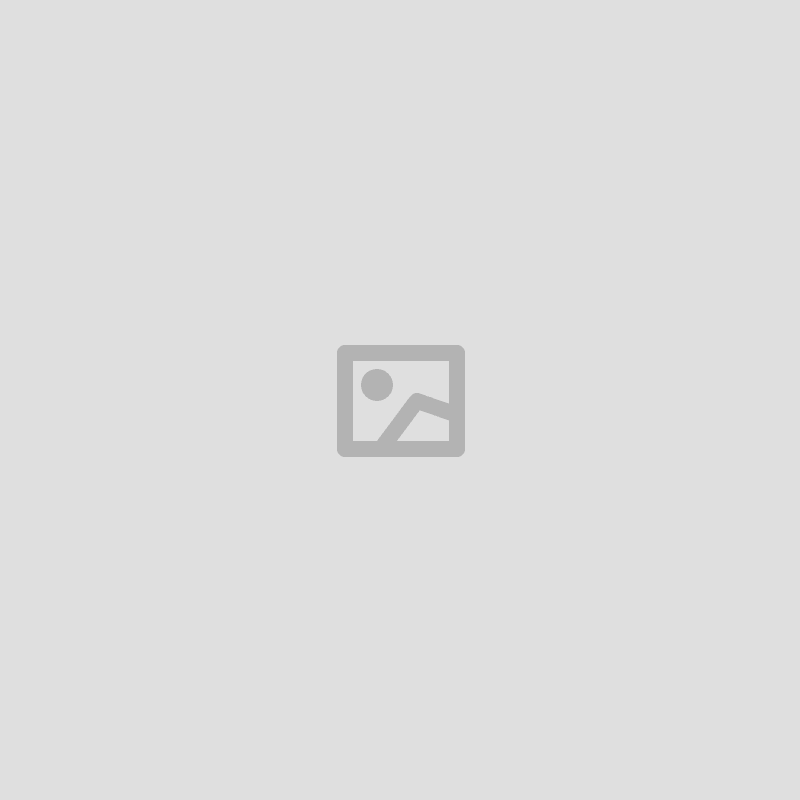
Ho bisogno che i post siano presenti invece del testo "i risultati della ricerca appariranno qui", poi quando avviene la ricerca, filtra semplicemente i risultati. È possibile fare questo con questo metodo? Attualmente ho implementato questa soluzione e funziona, ma ho una pagina vuota prima che avvenga la ricerca
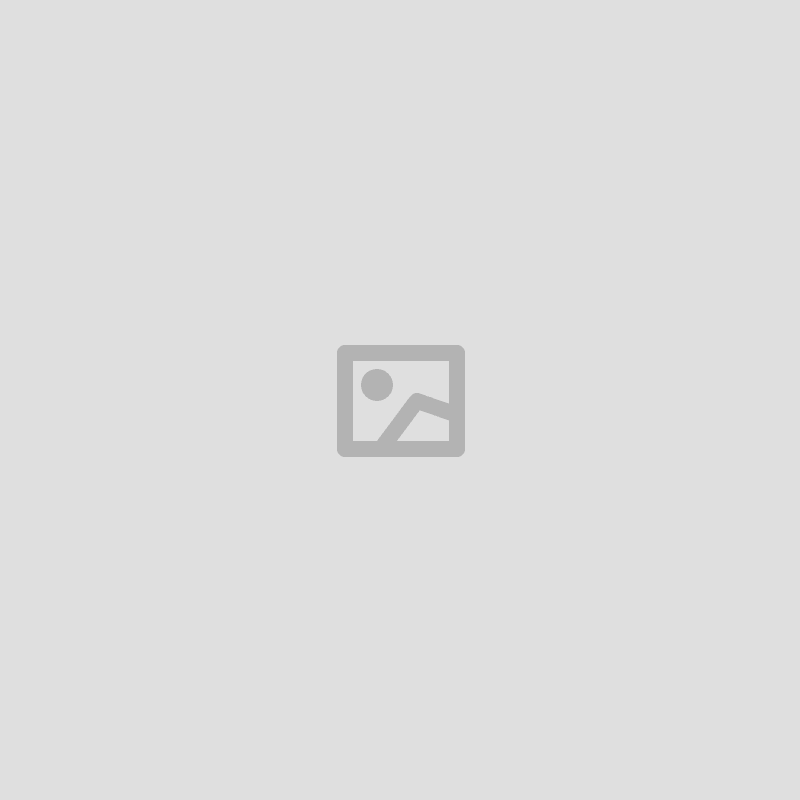
Hai dimenticato di chiamarlo come searchinput, puoi incollare il codice qui sotto in functions.php
// la funzione ajax
add_action('wp_ajax_data_fetch' , 'data_fetch');
add_action('wp_ajax_nopriv_data_fetch','data_fetch');
function data_fetch(){
$the_query = new WP_Query( array( 'posts_per_page' => 5, 's' => esc_attr( $_POST['keyword'] ), 'post_type' => 'post' ) );
if( $the_query->have_posts() ) :
while( $the_query->have_posts() ): $the_query->the_post(); ?>
<a href="<?php echo esc_url( post_permalink() ); ?>"><?php the_title();?></a>
<?php endwhile;
wp_reset_postdata();
else:
echo '<h3>Nessun risultato trovato</h3>';
endif;
die();
}
// aggiungi il js per il fetch ajax
add_action( 'wp_footer', 'ajax_fetch' );
function ajax_fetch() {
?>
<script type="text/javascript">
function fetchResults(){
var keyword = jQuery('#searchInput').val();
if(keyword == ""){
jQuery('#datafetch').html("");
} else {
jQuery.ajax({
url: '<?php echo admin_url('admin-ajax.php'); ?>',
type: 'post',
data: { action: 'data_fetch', keyword: keyword },
success: function(data) {
jQuery('#datafetch').html( data );
}
});
}
}
</script>
<?php
}
Una volta fatto, trova e apri searchform.php. Ora quello che devi fare è sostituire l'elemento id con quello sopra (searchInput) e aggiungi questo codice
onkeyup="fetchResults()"
Accanto all'elemento id, quindi il codice complessivo sarà così
<form method="get" class="td-search-form-widget" action="<?php echo esc_url(home_url( '/' )); ?>">
<div role="search">
<input class="td-widget-search-input" type="text" value="<?php echo get_search_query(); ?>" name="s" id="searchInput" onkeyup="fetchResults()" /><input class="wpb_button wpb_btn-inverse btn" type="submit" id="searchsubmit" value="<?php _etd('Cerca', TD_THEME_NAME)?>" />
</div>
</form>
Assicurati di fare le modifiche nel child theme.
Ora l'ultimo passo: aggiungi <div id="datafetch"></div>
appena sopra e potrai vedere la ricerca live nel tuo modulo di ricerca.
Puoi anche farlo tramite un plugin di ricerca live, il modo più semplice se non sai programmare. Spero sia utile.
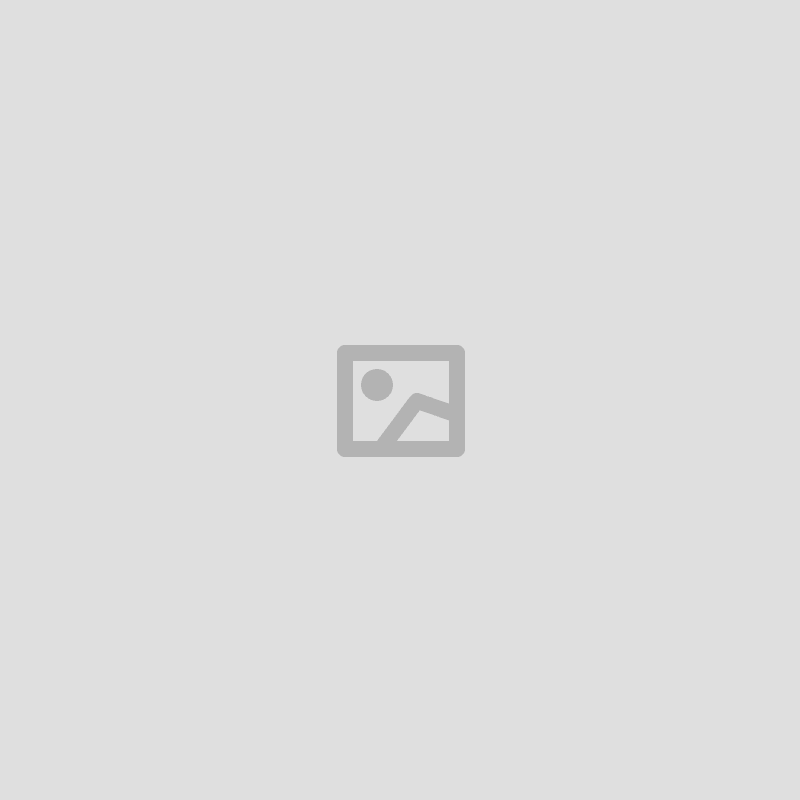
/* Campi aggiunti nel profilo admin */
add_action( 'show_user_profile', 'extra_user_profile_fields' );
add_action( 'edit_user_profile', 'extra_user_profile_fields' );
function extra_user_profile_fields( $user ) { ?>
<h3><?php _e("Informazioni aggiuntive del profilo", "blank"); ?></h3>
<table class="form-table">
<tr>
<th><label for="address"><?php _e("Indirizzo"); ?></label></th>
<td>
<input type="text" name="postSearch" id="postSearch" value="<?php echo esc_attr( get_the_author_meta( 'postSearch', $user->ID ) ); ?>" class="regular-text" /><br />
<span class="description"><?php _e("Inserisci il tuo indirizzo."); ?></span>
<div id="datafetch"></div>
</td>
</tr>
</table>
<?php }
// Chiamata jQuery Ajax qui
add_action( 'admin_footer', 'ajax_fetch' );
function ajax_fetch() {
?>
<script type="text/javascript">
(function($){
var searchRequest = null;
jQuery(function () {
var minlength = 3;
jQuery("#postSearch").keyup(function () {
var that = this,
value = jQuery(this).val();
if (value.length >= minlength ) {
if (searchRequest != null)
searchRequest.abort();
searchRequest = jQuery.ajax({
type: "POST",
url: "<?php echo admin_url('admin-ajax.php'); ?>",
data: {
action: 'data_fetch',
search_keyword : value
},
dataType: "html",
success: function(data){
//controlliamo se il valore è lo stesso
if (value==jQuery(that).val()) {
jQuery('#datafetch').html(data);
}
}
});
}
});
});
}(jQuery));
</script>
<?php
}
// funzione ajax
add_action('wp_ajax_data_fetch' , 'data_fetch');
add_action('wp_ajax_nopriv_data_fetch','data_fetch');
function data_fetch(){
$the_query = new WP_Query( array( 'posts_per_page' => -1, 's' => esc_attr( $_POST['search_keyword'] ), 'post_type' => 'post' ) );
if( $the_query->have_posts() ) :
while( $the_query->have_posts() ): $the_query->the_post(); ?>
<h2><a href="<?php echo esc_url( post_permalink() ); ?>"><?php the_title();?></a></h2>
<?php endwhile;
wp_reset_postdata();
endif;
die();
}
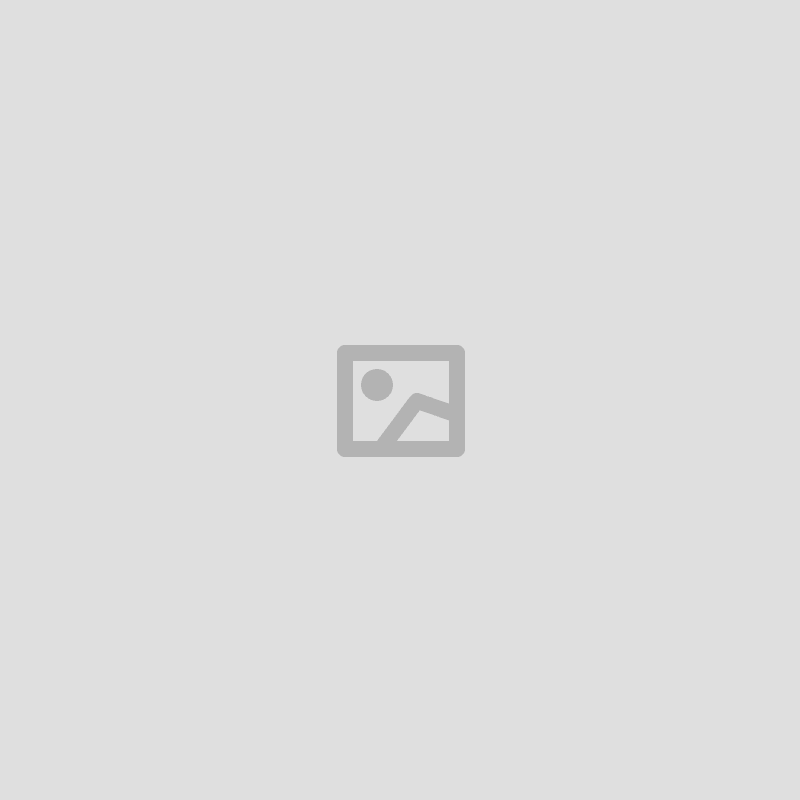
Mi dispiace menzionarlo (e non prenderlo come un attacco personale) ma per favore non rispondere suggerendo un plugin! So che gli utenti qui su wpse non possono aggiungere un commento finché non raggiungono un certo numero di punti reputazione. È anche noto che wpse è pensato per chiedere aiuto con il codice e non per trovare un plugin, per quello c'è il repository su WordPress.org. Peggio ancora, il tuo consiglio riguarda un plugin commerciale di terze parti. Secondo me non è corretto e segnalerò questa risposta.
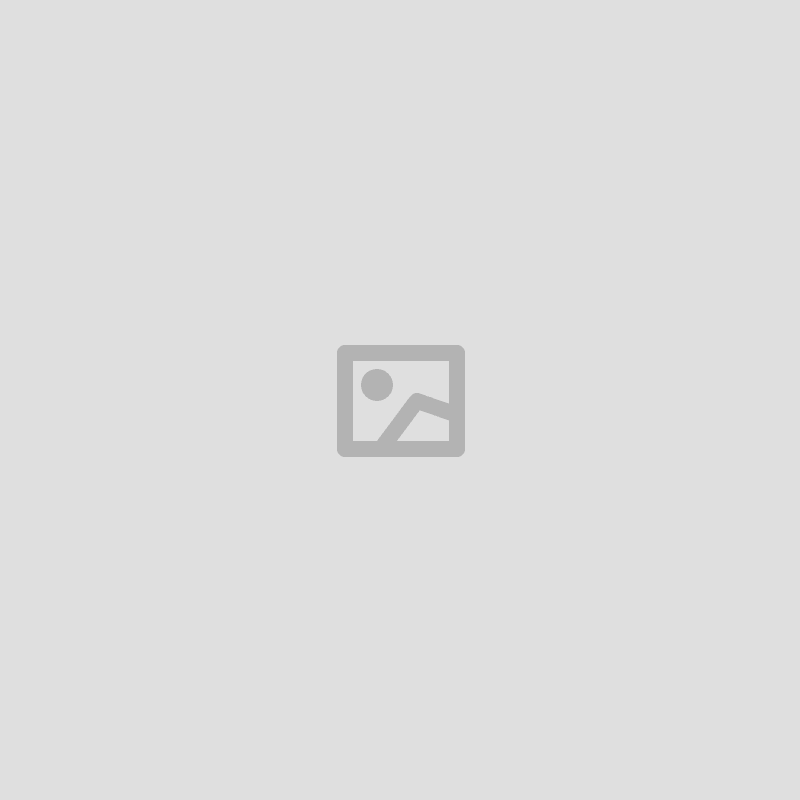