Ordinare articoli per nome categoria e titolo
Sto cercando di capire come posso visualizzare i miei articoli ordinati prima per titolo della categoria (a-z) e poi per il titolo degli articoli all'interno della categoria:
CATEGORIA A
- A post che inizia con a
- Because voglio essere mostrato per secondo
- Come on, mostrami già
CATEGORIA B
- Another post che inizia con a
- Bother, non riesco a trovare un altro titolo con B
- I guess hai capito l'idea
Come posso ottenere questo risultato?
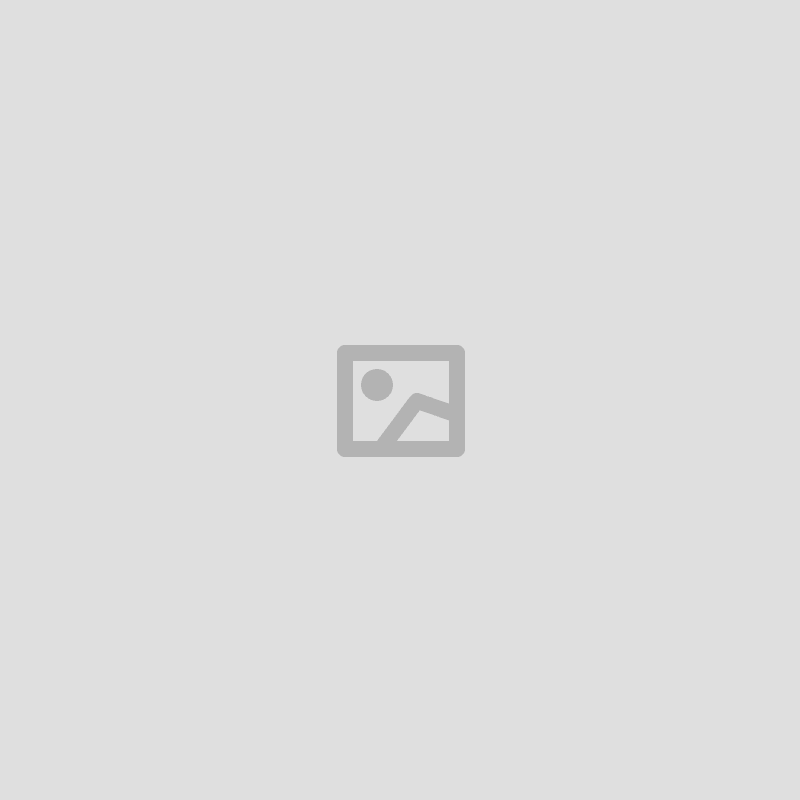
Per ottenere i post suddivisi per categoria, è necessario scorrere l'elenco delle categorie e poi effettuare una query per ogni categoria:
$categories = get_categories( array ('orderby' => 'name', 'order' => 'asc' ) );
foreach ($categories as $category) {
echo "Categoria: $category->name <br/>";
$catPosts = new WP_Query( array ( 'category_name' => $category->slug, 'orderby' => 'title' ) );
if ( $catPosts->have_posts() ) {
while ( $catPosts->have_posts() ) {
$catPosts->the_post();
echo "<a href='the_permalink()'>the_title()</a>";
}
echo "<p><a href='category/$category->slug'>Altro in questa categoria</a></p>";
} //fine if
} //fine foreach
wp_reset_postdata();
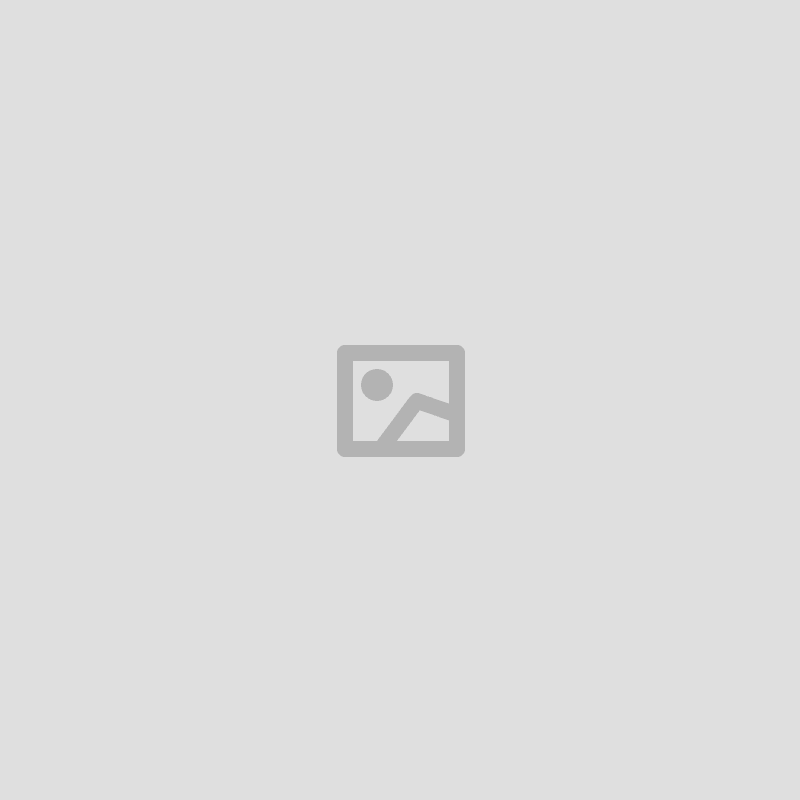
Per la scalabilità, non consiglio di utilizzare più elementi WP_Query
quando non sono necessari. Usare un singolo get_terms
e un singolo WP_Query
è tutto ciò di cui hai bisogno. Poi avrai tutti gli elementi e potrai usare array_filter
per capire quali articoli appartengono a ciascuna categoria.
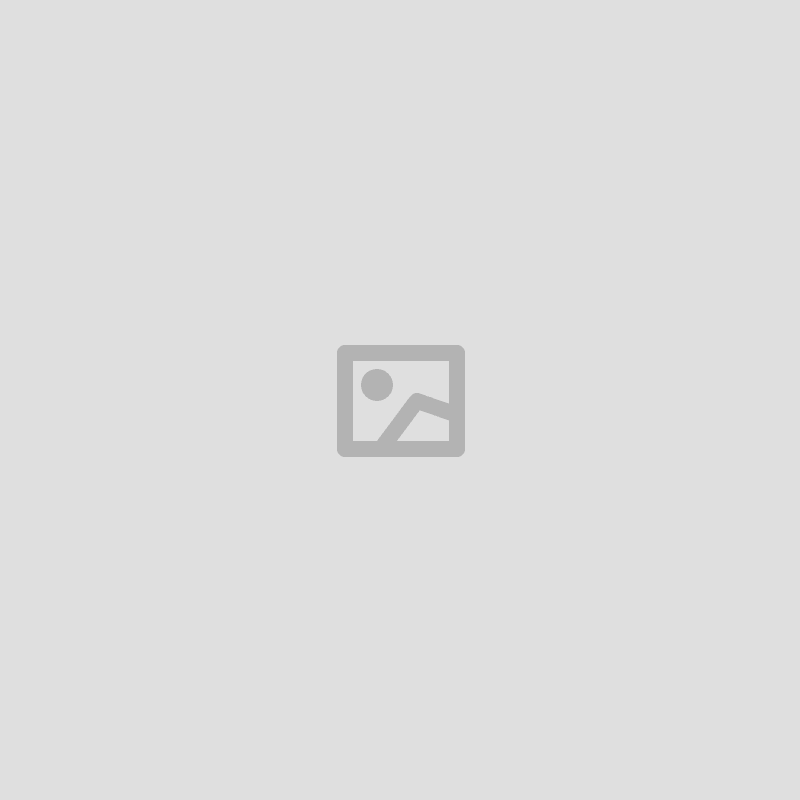
Penso che tu intenda GRUPPARE i Post
per categoria/tassonomia NON ORDINARLI
.
Ecco un codice per GRUPPARE
per categoria/tassonomia
$terms = get_terms( 'my_cat_name' );
Qui, cat_name
è il nome della tassonomia, quando la registri in questo modo: es.
register_taxonomy( 'my_cat_name', array( 'custom_post_name' ), $args )
Usalo nella
Query
es.:$args = array( 'post_type' => 'custom_post_name', 'my_cat_name' => $term->slug, 'posts_per_page' => $no_of_posts, );
Codice completo:
$terms = get_terms( 'CUSTOM_TAXONOMY_SLUG' ); if ( ! empty( $terms ) && ! is_wp_error( $terms ) ){ $output .= '<ul class="category-list">'; foreach ( $terms as $term ) { $output .= '<li class="single-cat">'; $output .= ' <h3>' . $term->name . '</h3>'; // Nome Tassonomia/Categoria $args = array( 'post_type' => 'POST_TYPE_SLUG', 'CUSTOM_TAXONOMY_SLUG' => $term->slug, 'posts_per_page' => -1, ); $the_query = new WP_Query( $args ); if ( $the_query->have_posts() ) { $output .= '<ul class="cat-post-list">'; while ( $the_query->have_posts() ) { $the_query->the_post(); $output .=' <li class="cat-single-post">'; $output .=' <h4><a href="'.get_the_permalink().'">' .get_the_title(). '</a></h4>'; $output .=' </li><!-- .cat-single-post -->'; } $output .= '</ul><!-- .cat-post-list -->'; } wp_reset_postdata(); $output .= '</li><!-- .single-cat-item -->'; } $output .= '</ul><!-- .category-list -->'; }
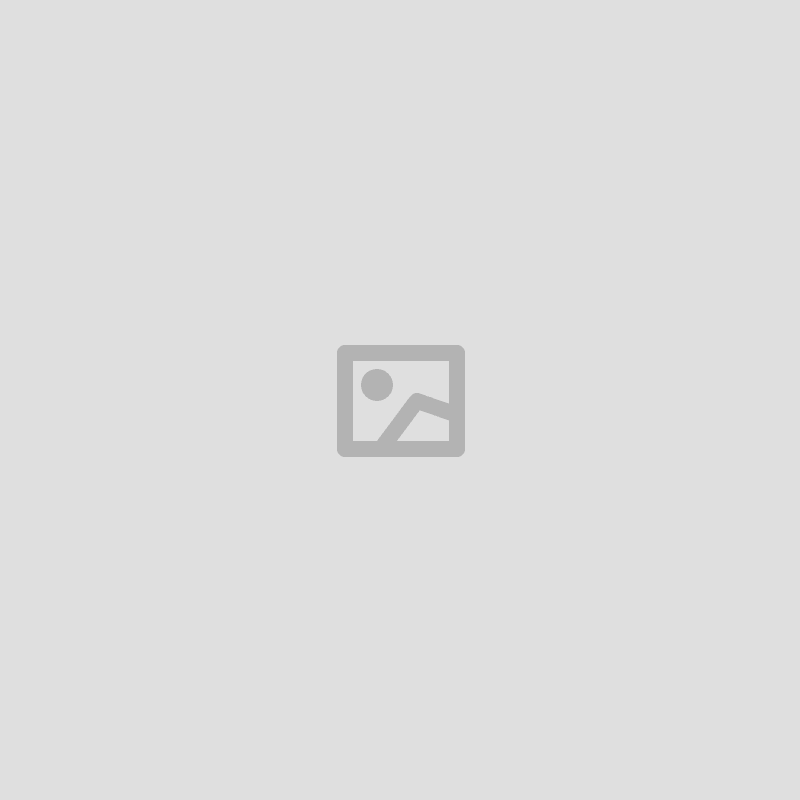
Espandendo il lavoro di Maheshwaghmare;
<?
$terms = get_terms( 'CUSTOM_TAXONOMY' );
if ( ! empty( $terms ) ){ ?>
<div class="POST_TYPE_PLURAL">
<? foreach ( $terms as $term ) {
//print_r($term) // DEBUG;
$term_slug = $term->slug;
$term_name = $term->name;
$term_description = $term->description;
?>
<div class="POST_TYPE_CATEGORY <?=$term_slug; ?>">
<h1 class="section-head"> <?=$term_name; ?> </h1>
<p><?=$term_description; ?> </p>
<?
$args = array(
'post_type' => 'CUSTOM_POST_TYPE',
'tax_query' => array(
array(
'taxonomy' => 'CUSTOM_TAXONOMY',
'field' => 'slug',
'terms' => $term_slug,
),
),
'posts_per_page' => -1,
);
$the_query = new WP_Query( $args );
if ( $the_query->have_posts() ) : ?>
<? while ( $the_query->have_posts() ) : $the_query->the_post(); ?>
<div class="POST_TYPE_SINGLE">
<h3> <? the_title(); ?> </h3>
<? // ALTRO CODICE DEL TEMPLATE ?>
</div>
<? endwhile;
endif;
wp_reset_postdata(); ?>
</div>
<? } // foreach
} //if terms
?>
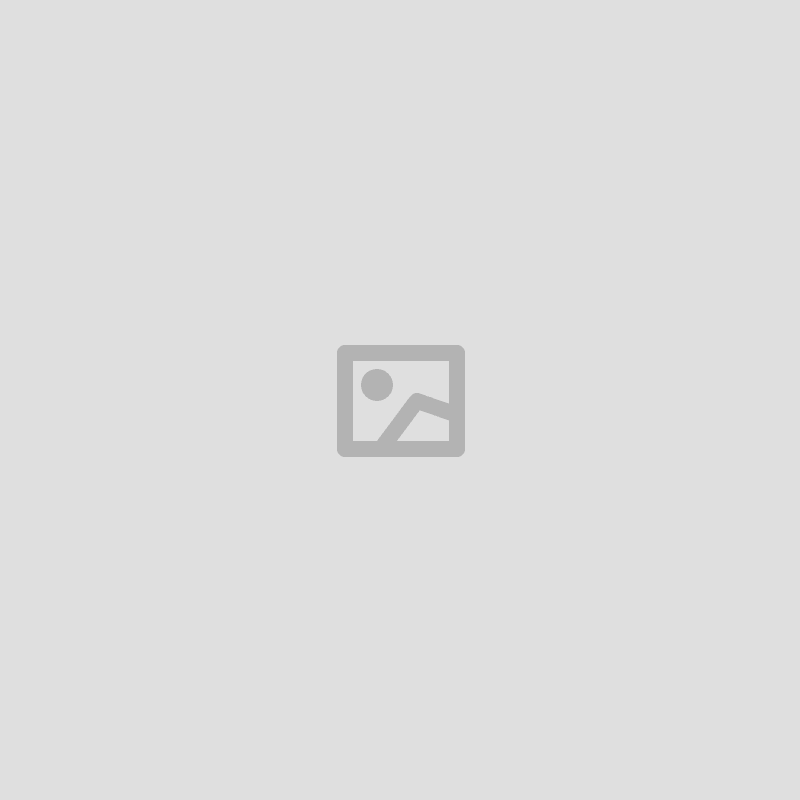
Sarei interessato a sapere se è meglio continuare a creare istanze di wp_query o se sarebbe meglio creare un array da una e poi usare l'array per popolare.
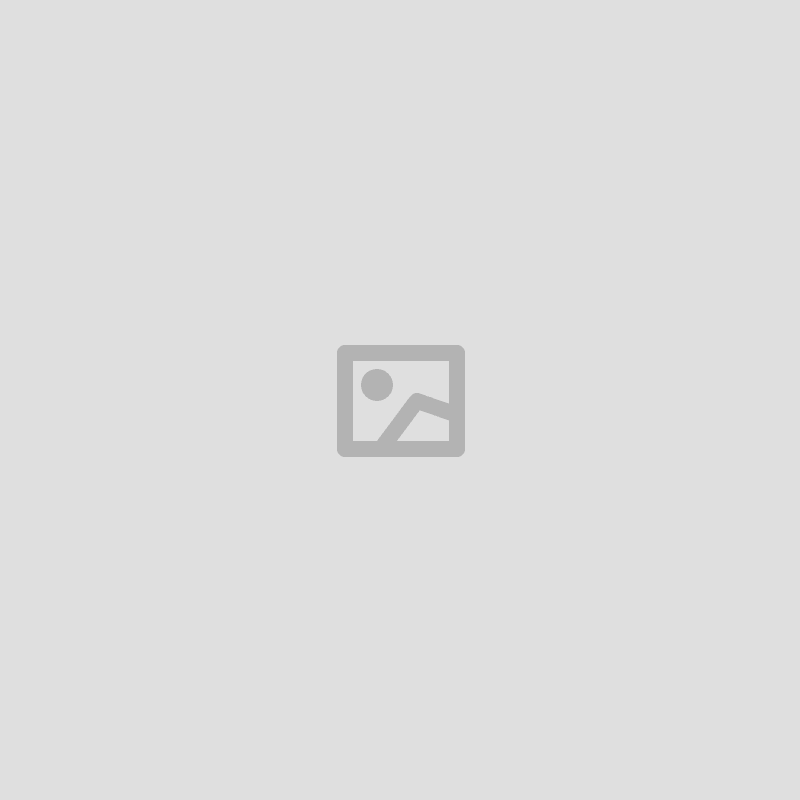
Puoi utilizzare il parametro orderby
per una nuova istanza di wp_query
:
$query = new WP_Query( array ( 'orderby' => 'title', 'order' => 'DESC' ) );
Quindi per ogni categoria utilizza un'istanza separata.
Maggiori informazioni qui: http://codex.wordpress.org/Class_Reference/WP_Query
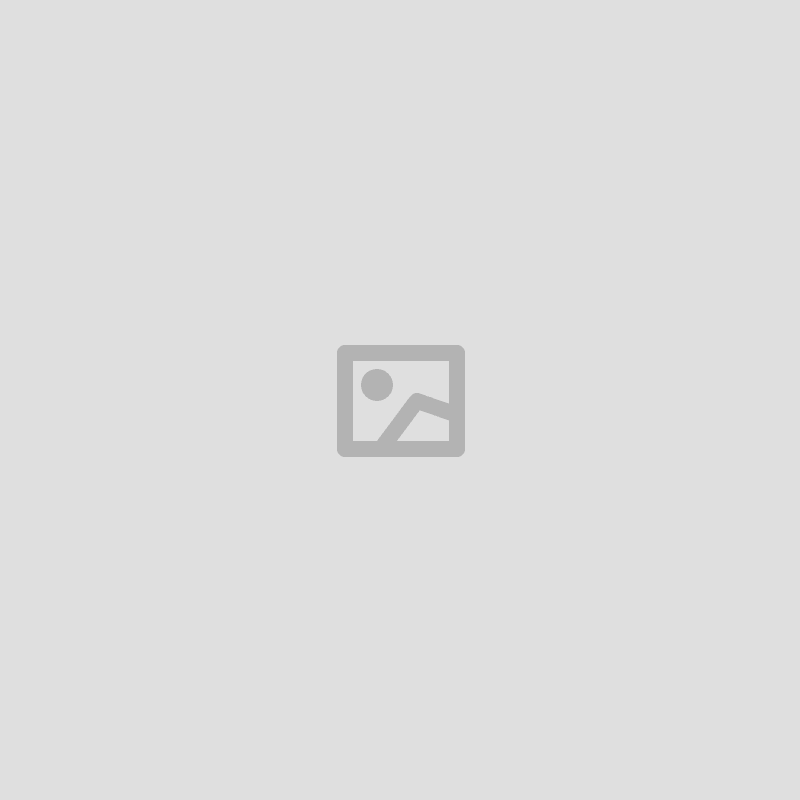