Verifica se il Titolo del Post esiste, Inserisci il post se non esiste, Aggiungi Numero Incrementale alla Meta se esiste
Ho già una funzione dove un utente invia un form e crea un post personalizzato...
<?php
// Ottieni il titolo del post e il valore del submit
$postTitle = $_POST['post_title'];
$submit = $_POST['submit'];
if(isset($submit)){
global $user_ID;
// Verifica se esiste già un post con lo stesso titolo
$existing_post = get_page_by_title($postTitle, OBJECT, 'stuff');
if($existing_post === null) {
// Crea nuovo post se il titolo non esiste
$new_post = array(
'post_title' => $postTitle,
'post_content' => '',
'post_status' => 'publish',
'post_date' => date('Y-m-d H:i:s'),
'post_author' => '',
'post_type' => 'stuff',
'post_category' => array(0)
);
$post_id = wp_insert_post($new_post);
add_post_meta($post_id, 'times', '1');
} else {
// Incrementa il contatore meta se il post esiste
$times = get_post_meta($existing_post->ID, 'times', true);
$new_times = intval($times) + 1;
update_post_meta($existing_post->ID, 'times', $new_times);
}
}
Voglio verificare se il titolo del post personalizzato esiste, quindi se NON esiste, procedere e creare il post con un #1 nel campo meta, e se esiste, semplicemente aggiungere 1 al campo meta
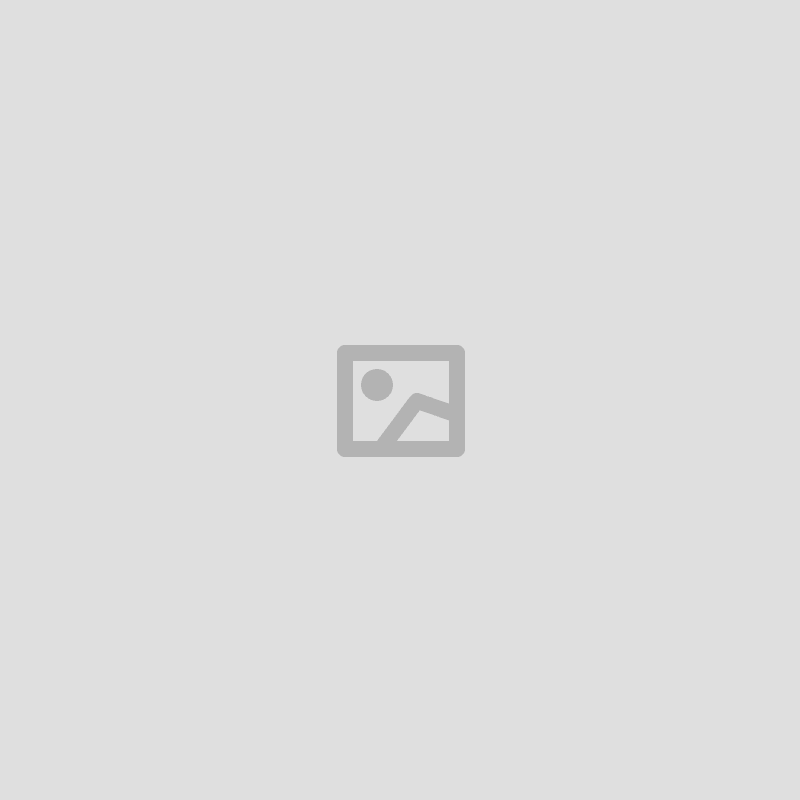
Un metodo più aggiornato potrebbe utilizzare la funzione post_exists()
in questo modo:
if( isset( $_POST['submit'] ) ){
$post_title = sanitize_title( $_POST['post_title'] );
$new_post = array(
'post_title' => $post_title,
'post_content' => '',
'post_status' => 'publish',
'post_date' => date('Y-m-d H:i:s'),
'post_author' => '',
'post_type' => 'stuff',
'post_category' => array(0)
);
$post_id = post_exists( $post_title ) or wp_insert_post( $new_post );
update_post_meta( $post_id, 'times', '1' );
}
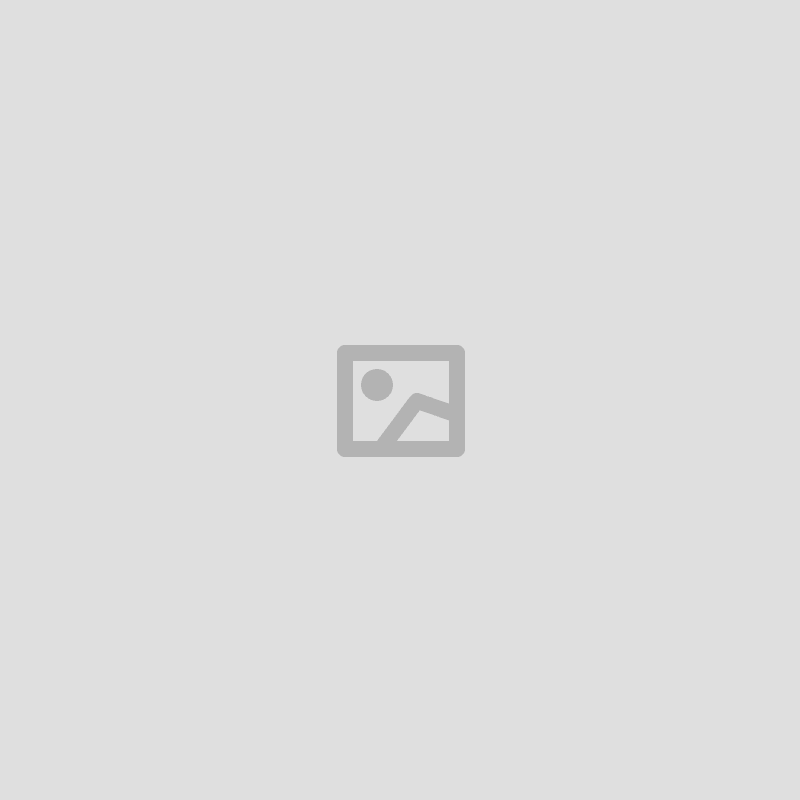
Non è del tutto chiaro se l'OP volesse incrementare il campo meta di 1 se il post esiste, o semplicemente impostare il campo meta a 1. Il codice sopra lo imposterà sempre a 1. Per incrementarlo, l'operatore ternario $post_id = post_exists[...]
dovrebbe essere scomposto in un if / else per poter incrementare il campo meta.
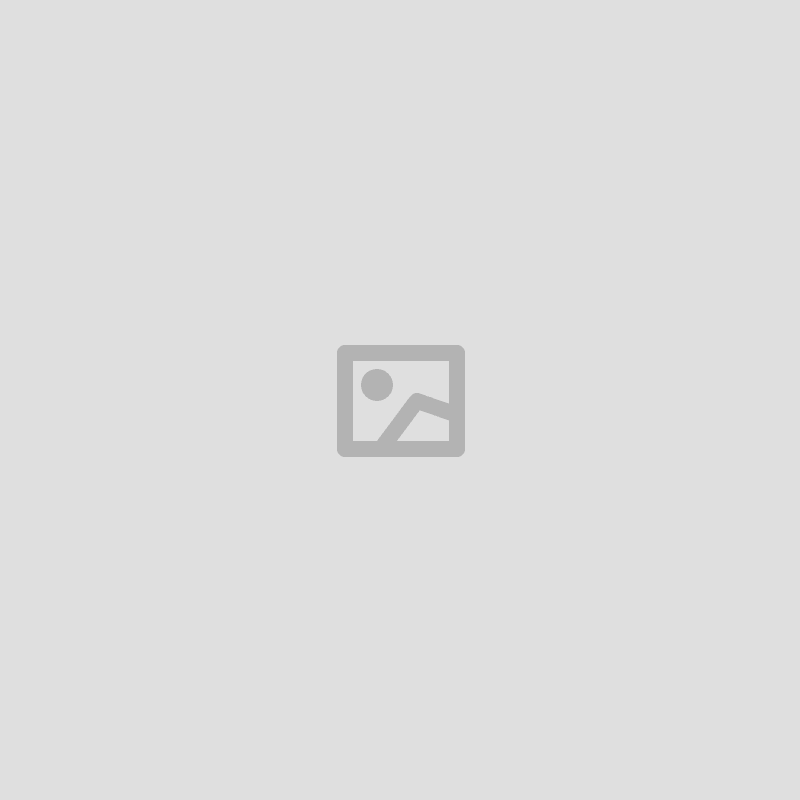
Questo richiederebbe una query.
Quindi basandosi sul tuo codice:
<?php
$postTitle = $_POST['post_title'];
$submit = $_POST['submit'];
if(isset($submit)){
global $user_ID, $wpdb;
$query = $wpdb->prepare(
'SELECT ID FROM ' . $wpdb->posts . '
WHERE post_title = %s
AND post_type = \'stuff\'',
$postTitle
);
$wpdb->query( $query );
if ( $wpdb->num_rows ) {
$post_id = $wpdb->get_var( $query );
$meta = get_post_meta( $post_id, 'times', TRUE );
$meta++;
update_post_meta( $post_id, 'times', $meta );
} else {
$new_post = array(
'post_title' => $postTitle,
'post_content' => '',
'post_status' => 'publish',
'post_date' => date('Y-m-d H:i:s'),
'post_author' => '',
'post_type' => 'stuff',
'post_category' => array(0)
);
$post_id = wp_insert_post($new_post);
add_post_meta($post_id, 'times', '1');
}
}
Dovrebbe funzionare
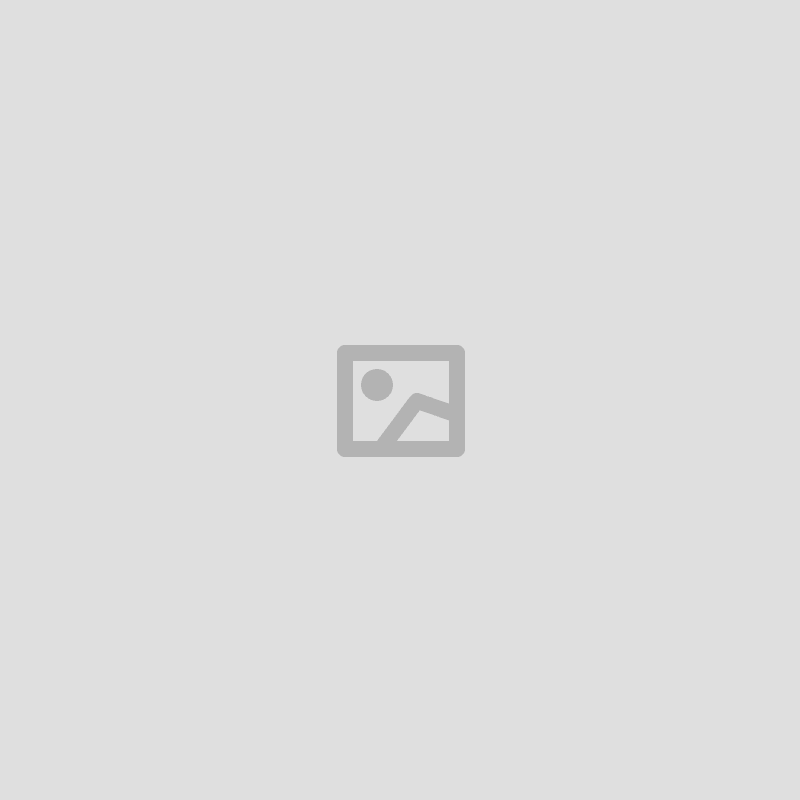
Potresti voler aggiungere AND post_status = 'publish'
alla query iniziale per restituire solo i post pubblicati.
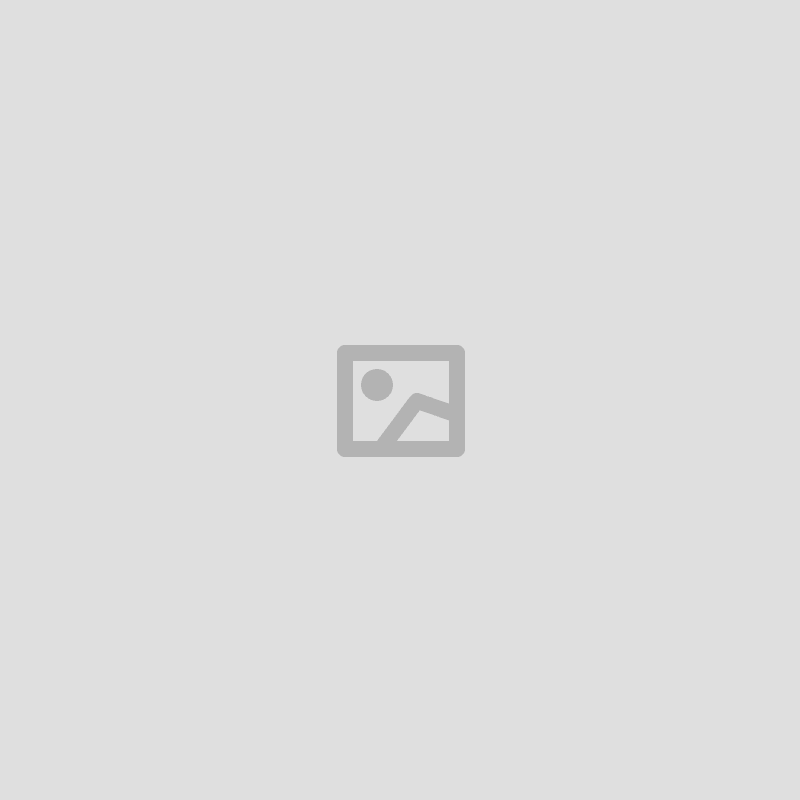
Grazie ancora per l'aiuto! Tuttavia, per qualche motivo i post non arrivano nel database.. né quelli nuovi né quelli vecchi aggiornati
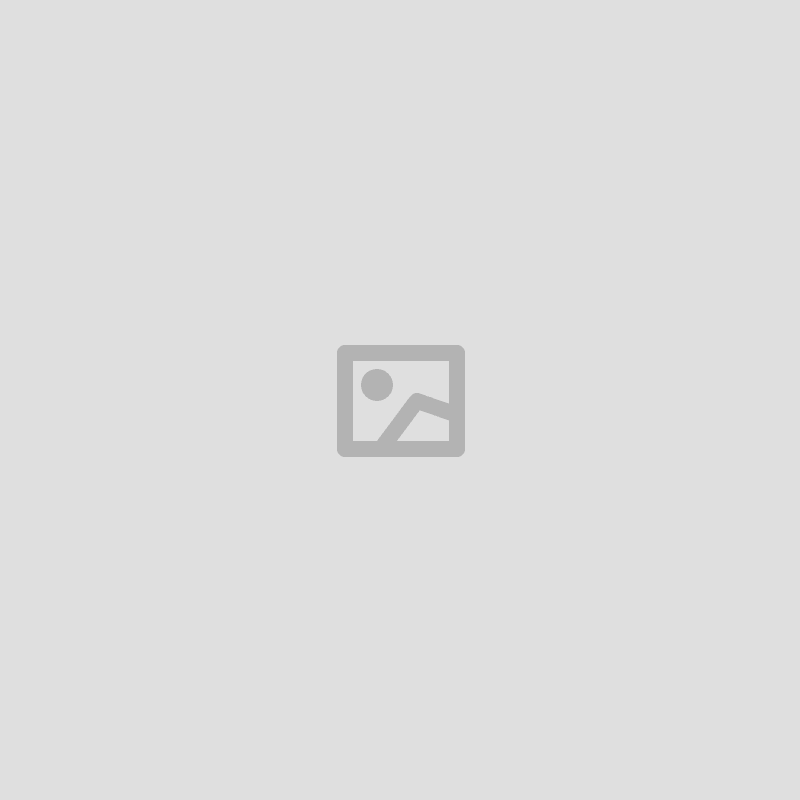
Fallimento epico da parte mia WHERE post_title = %d
dovrebbe essere WHERE post_title = %s
sbatto la testa sulla scrivania
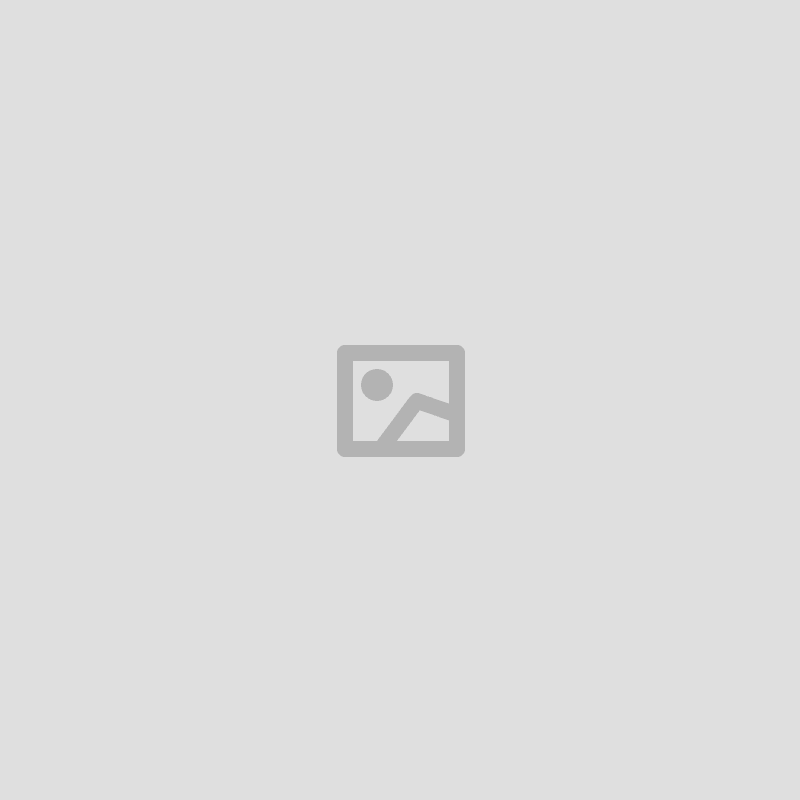
Succede anche ai migliori! Ok, quindi i nuovi post stanno venendo inseriti ora, ma il meta value per "times" non si incrementa. Viene invece creato un nuovo meta value chiamato "2". Non si auto-incrementa neanche quello.
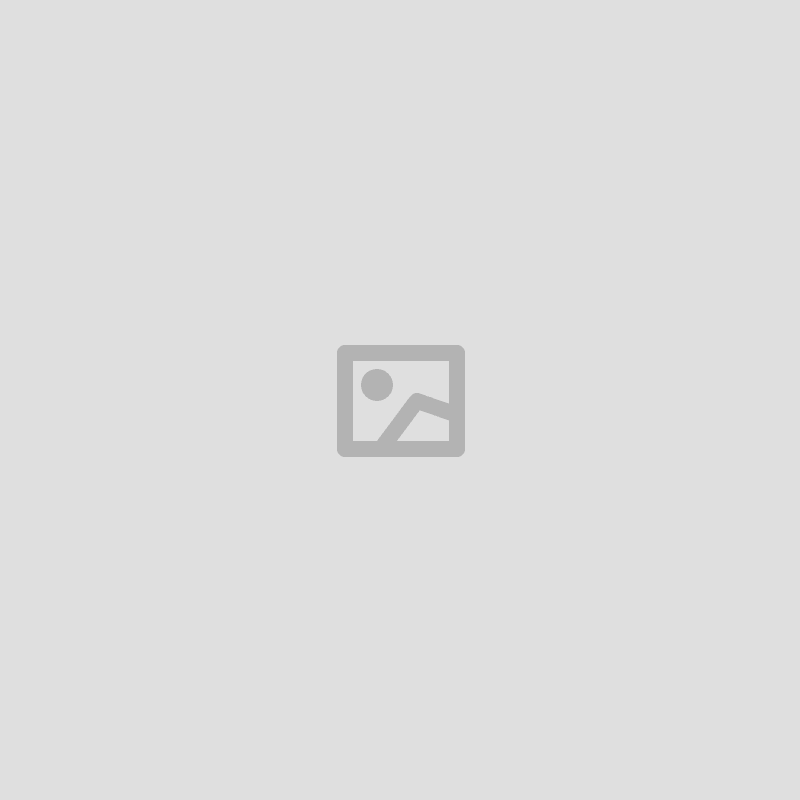
Ops, ho dimenticato di aggiornare anche quella riga. update_post_meta( $post_id, $meta );
dovrebbe essere update_post_meta( $post_id, 'times', $meta );
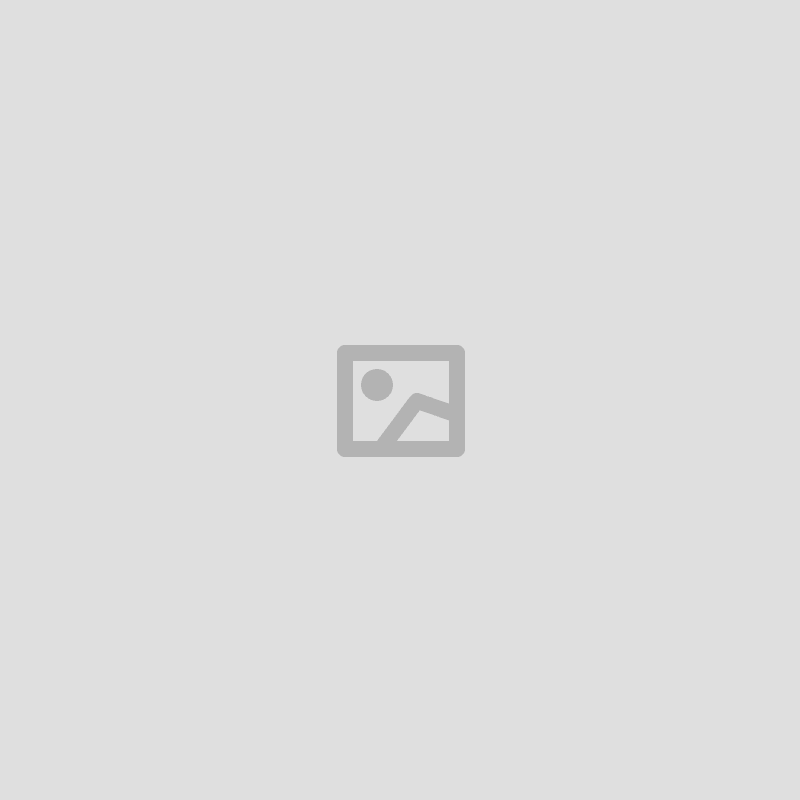
Puoi utilizzare la funzione get_page_by_title() di WordPress:
<?php $postTitle = $_POST['post_title'];
$submit = $_POST['submit'];
if(isset($submit)){
// Cerca un post personalizzato per titolo
$customPost = get_page_by_title($postTitle, OBJECT, 'stuff');
if(!is_null($customPost)) {
// Se il post esiste, incrementa il meta valore 'times'
$meta = get_post_meta($customPost->ID, 'times', true);
$meta++;
update_post_meta($customPost->ID, 'times', $meta);
return
}
global $user_ID;
// Crea un nuovo post personalizzato
$new_post = array(
'post_title' => $postTitle, // Titolo del post
'post_content' => '', // Contenuto vuoto
'post_status' => 'publish', // Stato pubblicato
'post_date' => date('Y-m-d H:i:s'), // Data corrente
'post_author' => '', // Autore vuoto
'post_type' => 'stuff', // Tipo di post personalizzato
'post_category' => array(0) // Nessuna categoria
);
// Inserisci il nuovo post e aggiungi il meta valore 'times'
$post_id = wp_insert_post($new_post);
add_post_meta($post_id, 'times', '1');
}
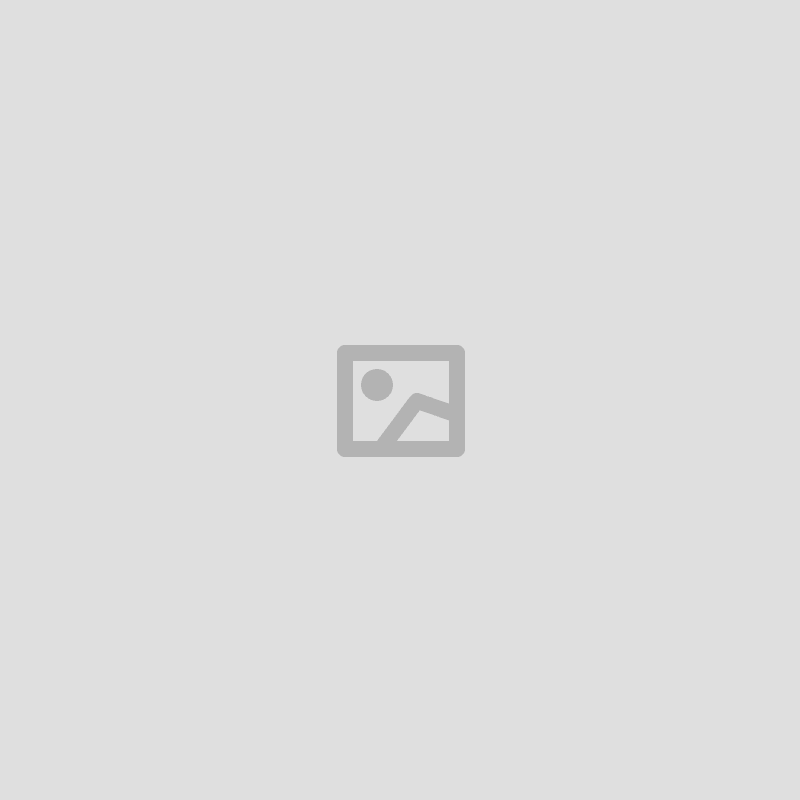
Ciao Alex, benvenuto su WPSE. Sentiti libero di fare il tour. Qui seguiamo il principio di insegnare a pescare piuttosto che limitarci a regalare il pesce. Potresti modificare il tuo post per aggiungere una spiegazione sul perché questa soluzione risolve il problema dell'OP?
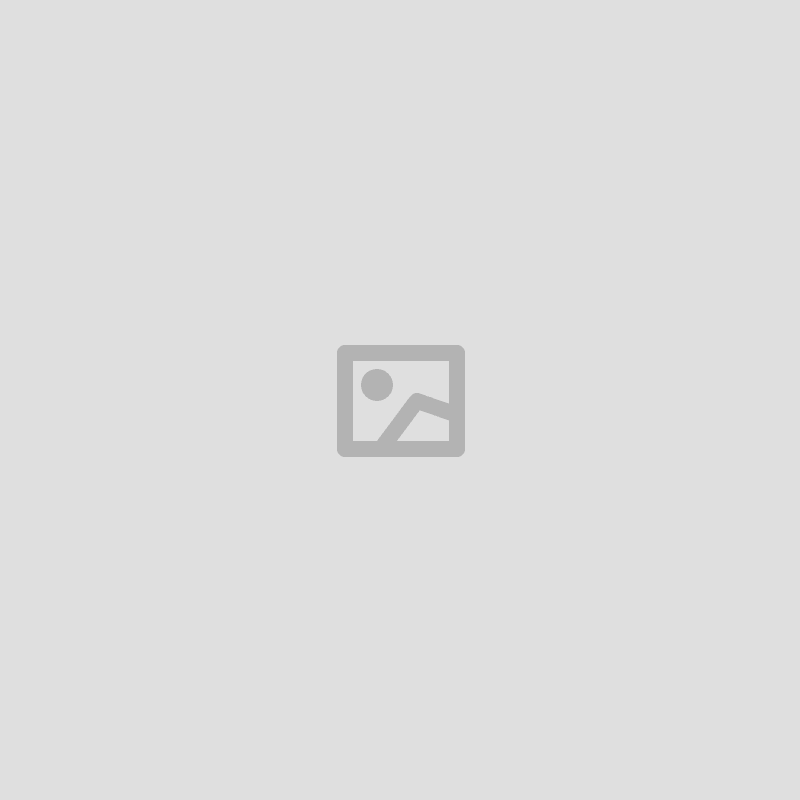
@Tim Hallman ottima risposta. Usa la funzione post_exists() per verificare se un post esiste o meno. Per maggiori dettagli visita https://developer.wordpress.org/reference/
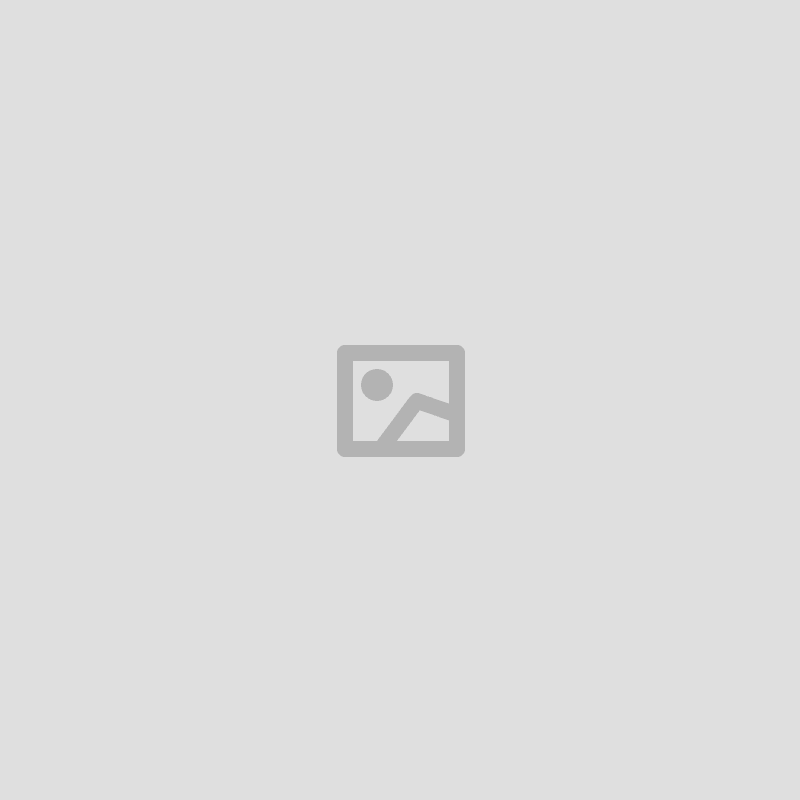
Puoi farlo tramite ID
$post_title = "Questo Titolo Fantastico";
$post_content = "Il mio contenuto di qualcosa di interessante.";
$post_status = "publish"; //publish, draft, etc
$post_type = "page" // o qualsiasi tipo di post desiderato
/* Tentativo di trovare l'ID del post tramite il titolo se esiste */
$found_post_title = get_page_by_title( $post_title, OBJECT, $post_type );
$found_post_id = $found_post_title->ID;
/**********************************************************
** Controlla se la pagina non esiste, se vero, crea un nuovo post
************************************************************/
if ( FALSE === get_post_status( $found_post_id ) ):
$post_args = array(
'post_title' => $post_title,
'post_type' => $post_type,
'post_content'=> $post_content,
'post_status' => $post_status,
//'post_author' => get_current_user_id(),
/* Se hai campi meta in cui inserire dati */
'meta_input' => array(
'meta_key1' => 'il mio valore',
'meta_key2' => 'il mio altro valore',
),
);
/* Aggiungi un nuovo post wp nel database, restituisci il suo ID */
$returned_post_id = wp_insert_post( $post_args );
/* Aggiorna il template della pagina solo se usi "page" come post_type */
update_post_meta( $returned_post_id, '_wp_page_template', 'my-page-template.php' );
/* Aggiungi valori nei campi meta. Funziona con ACF CUSTOM FIELDS!! */
$field_key = "My_Field_KEY";
$value = "il mio valore personalizzato";
update_field( $field_key, $value, $returned_post_id );
$field_key = "My_Other_Field_KEY";
$value = "il mio altro valore personalizzato";
update_field( $field_key, $value, $returned_post_id );
/* Salva un valore di checkbox o select */
// $field_key = "My_Field_KEY";
// $value = array("rosso", "blu", "giallo");
// update_field( $field_key, $value, $returned_post_id );
/* Salva in un campo repeater */
// $field_key = "My_Field_KEY";
// $value = array(
// array(
// "ss_name" => "Foo",
// "ss_type" => "Bar"
// )
// );
// update_field( $field_key, $value, $returned_post_id );
/* Mostra una risposta! */
echo "<span class='pg-new'><strong>". $post_title . " Creato!</strong></span><br>";
echo "<a href='".esc_url( get_permalink($returned_post_id) )."' target='_Blank'>". $post_title . "</a><p>";
else:
/***************************
** SE IL POST ESISTE, aggiornalo
****************************/
/* Aggiorna il post */
$update_post_args = array(
'ID' => $found_post_id,
'post_title' => $post_title,
'post_content' => $post_content,
);
/* Aggiorna il post nel database */
wp_update_post( $update_post_args );
/* Aggiorna i valori nei campi meta */
$field_key = "My_Field_KEY";
$value = "il mio valore personalizzato";
update_field( $field_key, $value, $found_post_id );
$field_key = "My_Other_Field_KEY";
$value = "il mio altro valore personalizzato";
update_field( $field_key, $value, $found_post_id );
/* Mostra una risposta! */
echo "<span class='pg-update'><strong>". $post_title . " Aggiornato!</strong></span><br>";
echo "<a href='".esc_url( get_permalink($found_post_id) )."' target='_Blank'>Visualizza</a> | <a href='post.php?post=".$found_post_id."&action=edit'>". $post_title . "</a><p>";
endif;
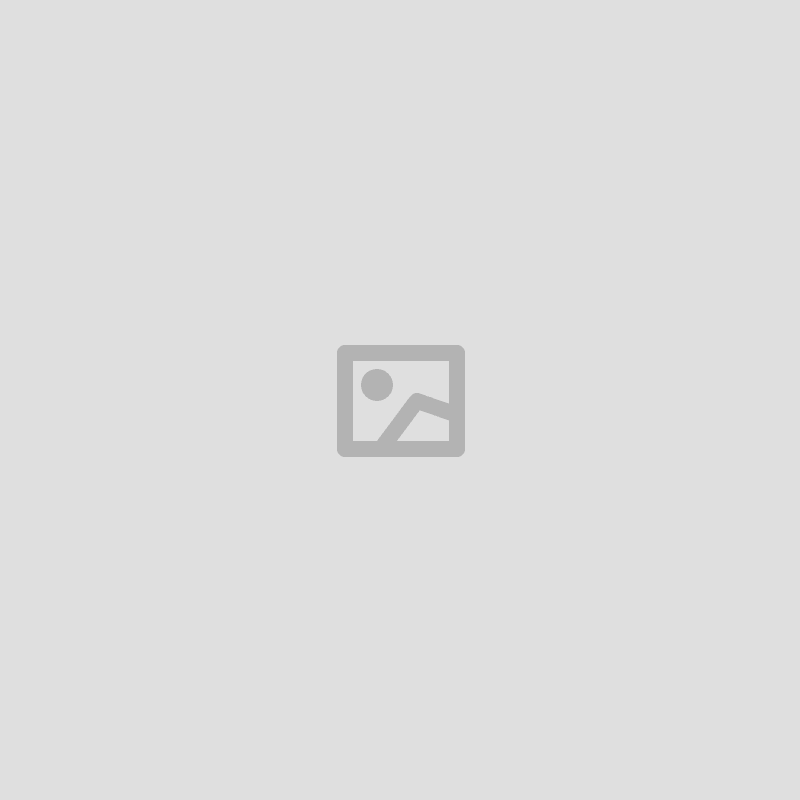
In realtà è più sicuro utilizzare le 2 funzioni integrate di WP progettate per questo scopo, post_exists
e wp_insert_post
come nella risposta di @TimHallman. Più codice non necessario introduci, maggiore è la possibilità di introdurre errori o problemi di manutenzione a lungo termine.
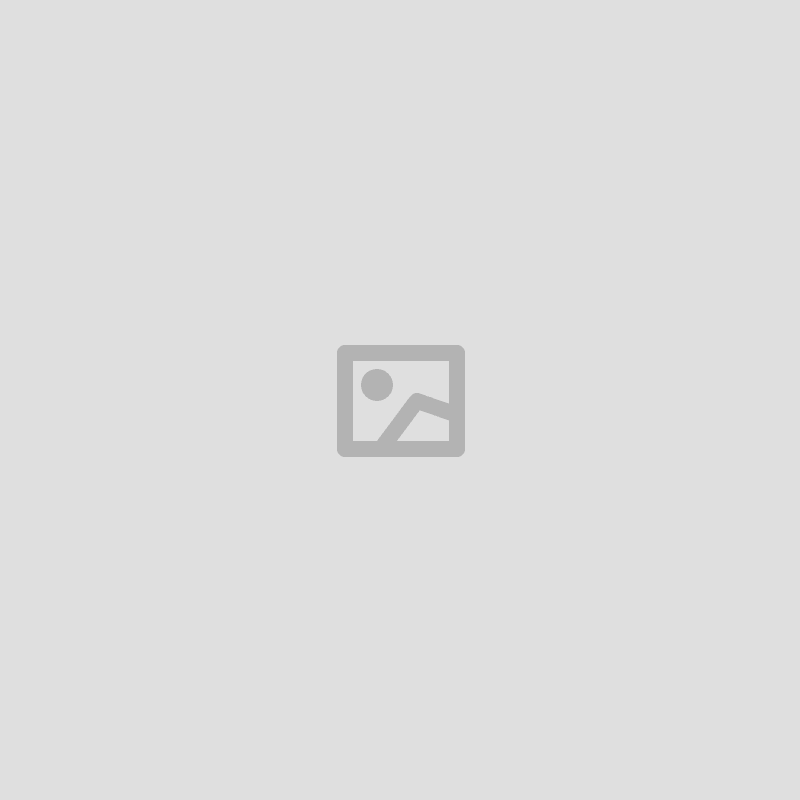
WordPress verifica se un post esiste per titolo
function wp_exist_post_by_title( $title ) {
global $wpdb;
$return = $wpdb->get_row( "SELECT ID FROM wp_posts WHERE post_title = '" . $title . "' && post_status = 'publish' && post_type = 'post' ", 'ARRAY_N' );
if( empty( $return ) ) {
return false;
} else {
return true;
}
}
// utilizzo
if( wp_exist_post_by_title( $post->name ) ) {
// il post esiste
} else {
// il post non esiste
}
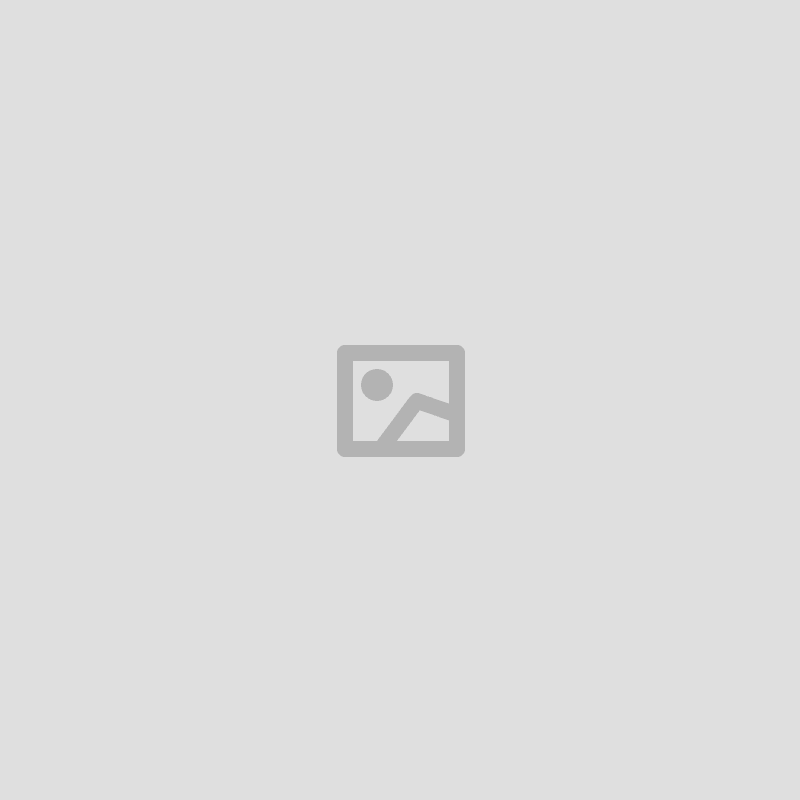